--- gid: d80f05f7-bb04-4fe9-be8b-d997247516c1 title: Add a banner to checkout description: Learn how to use checkout UI extension settings to add a custom banner to checkout. --- import Deploy from 'app/views/partials/extensions/deploy.mdx' import CheckoutUiRequirements from 'app/views/partials/apps/checkout/ui-extensions/requirements.mdx' import CheckoutUiCreate from 'app/views/partials/apps/checkout/ui-extensions/create.mdx' import CheckoutUiPreview from 'app/views/partials/apps/checkout/ui-extensions/preview.mdx' import CheckoutUiReference from 'app/views/partials/apps/checkout/ui-extensions/reference.mdx' <Repo extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react" /> <Repo extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js" /> <Picker name="extension"> <PickerOption name="react" /> <PickerOption name="javascript" /> </Picker> <Overview> A custom banner is a notice that you can display to customers. For example, you might want to show a banner that indicates that items are final sale and can't be returned or exchanged. In this tutorial, you'll use [checkout UI extensions](/docs/api/checkout-ui-extensions) to add a customizable banner. Follow along with this tutorial to build a sample app, or clone the completed sample app. <Notice type="shopifyPlus" title="Shopify Plus"> Checkout UI extensions are available only to [Shopify Plus](https://www.shopify.com/plus) merchants. </Notice> 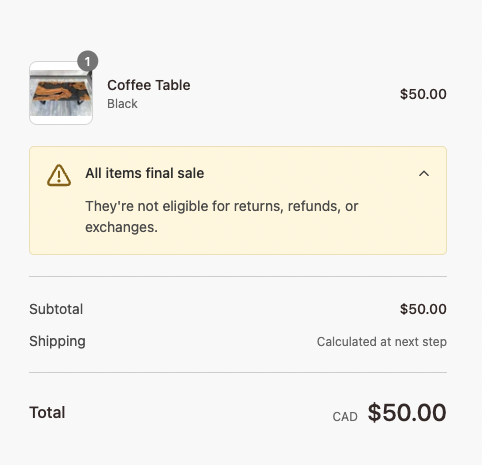 ## What you'll learn In this tutorial, you'll learn how to do the following: - Generate a checkout UI extension that appears in the checkout flow using Shopify CLI. - Set up a banner to display to customers. - Configure settings that enable app users to control the banner content. - Support multiple targets that enable app users to choose where to render the banner. - Deploy your extension code to Shopify. </Overview> <Requirements> <CheckoutUiRequirements /> </Requirements> <StepSection> <Step> ### Create a Checkout UI extension To create a checkout UI extension, use Shopify CLI, which generates starter code for building your extension. <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react/blob/main/extensions/custom-banner/src/Checkout.jsx" /> <CodeRef extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js/blob/main/extensions/custom-banner/src/Checkout.js" /> <CheckoutUiCreate /> </Substep> </Step> <Step> ### Set up a target for your extension Set up a target for your checkout UI extension. [Targets](/docs/api/checkout-extensions/checkout#extension-targets) control where your extension renders in the checkout flow. <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react/blob/main/extensions/custom-banner/src/Checkout.jsx" tag="custom-banner.ext-point"/> <CodeRef extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js/blob/main/extensions/custom-banner/src/Checkout.js" tag="custom-banner.ext-point"/> #### Export the targets from your script file In your <If extension="react">`Checkout.jsx`</If><If extension="javascript">`Checkout.js`</If> file, set the entrypoints for the checkout extension, and then export them so they can be referenced in your configuration. For each target that you want to use, create <If extension="react"> a `reactExtension`</If> <If extension="javascript">an `extension`</If> function that references your target, and export it using a named export. --- <If extension="react"> <Resources> [purchase.checkout.block.render](/docs/api/checkout-ui-extensions/latest/targets/block/purchase-checkout-block-render) [purchase.checkout.delivery-address.render-before](/docs/api/checkout-ui-extensions/latest/targets/shipping/purchase-checkout-delivery-address-render-before) </Resources> </If> <If extension="javascript"> <Resources> [purchase.checkout.block.render](/docs/api/checkout-ui-extensions/latest/targets/block/purchase-checkout-block-render) </Resources> </If> </Substep> <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react/blob/main/extensions/custom-banner/shopify.extension.toml" tag="custom-banner.config-ext-point"/> <CodeRef extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js/blob/main/extensions/custom-banner/shopify.extension.toml" tag="custom-banner.config-ext-point"/> <CheckoutUiReference /> </Substep> </Step> <Step> ### Configure the settings The settings for a checkout UI extension define fields that the app user can set from the [checkout editor](/docs/apps/build/checkout/test-checkout-ui-extensions#test-the-extension-in-the-checkout-editor). You can use validation options to apply additional constraints to the data that the setting can store, such as a minimum or maximum value. <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react/blob/main/extensions/custom-banner/shopify.extension.toml" tag="custom-banner.req-properties" /> <CodeRef extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js/blob/main/extensions/custom-banner/shopify.extension.toml" tag="custom-banner.req-properties" /> #### Define the required properties for the settings Define the settings that you want to expose to app users in [shopify.extension.toml](/docs/api/checkout-ui-extensions/latest/configuration). For each setting, define the [required properties](/docs/api/checkout-ui-extensions/latest/configuration#settings-definition): `key`, `type`, and `name`. --- The `key` property defines a string that's used to access the setting values from your extension code. The `type` property determines the type of information that the setting can store. Each setting types has built-in validation on the setting input. The `name` property defines the name for the setting that's displayed to the app user in the [checkout editor](/docs/apps/build/checkout/test-checkout-ui-extensions#test-the-extension-in-the-checkout-editor). </Substep> <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react/blob/main/extensions/custom-banner/shopify.extension.toml" tag="custom-banner.optional-properties" /> <CodeRef extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js/blob/main/extensions/custom-banner/shopify.extension.toml" tag="custom-banner.optional-properties" /> #### Define the optional properties for the settings For each setting, define the [optional properties](/docs/api/checkout-ui-extensions/latest/configuration#settings-definition): `description` and `validations`. --- The `description` property is displayed to the app user in the [checkout editor](/docs/apps/build/checkout/test-checkout-ui-extensions#test-the-extension-in-the-checkout-editor). The `validations` property defines [constraints](/docs/api/checkout-ui-extensions/latest/configuration#settings-definition) on the setting input that Shopify validates. </Substep> </Step> <Step> ### Use the configured settings in the UI extension Use the values that have been configured by the app user in the checkout UI extension. <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react/blob/main/extensions/custom-banner/src/Checkout.jsx" tag="custom-banner.use-settings" /> <CodeRef extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js/blob/main/extensions/custom-banner/src/Checkout.js" tag="custom-banner.use-settings" /> #### Access app user settings Retrieve the settings values within your extension. Set default settings so the app can be previewed without being deployed. <If extension="react"> In React, the `useSettings` hook re-renders your extension with the latest values. </If> <If extension="javascript"> Subscribe to changes in the settings and update your UI directly. </If> --- When an extension is being installed in the checkout editor, the settings are empty until an app user sets a value. This object is updated in real time as the app user fills in the settings. <If extension="react"> <Resources> [useSettings](/docs/api/checkout-ui-extensions/latest/react-hooks/storage/usesettings) </Resources> </If> </Substep> </Step> <Step> ### Render the banner Render the Checkout UI extension `Banner` component with the content from the settings that you configured. <Substep> #### Add the Banner component <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--custom-banner--react/blob/main/extensions/custom-banner/src/Checkout.jsx" tag="custom-banner.render"/> <CodeRef extension="javascript" href="https://github.com/Shopify/example-checkout--custom-banner--js/blob/main/extensions/custom-banner/src/Checkout.js" tag="custom-banner.render"/> Using the `Banner` component, create the custom banner. --- <Resources> [Banner](/docs/api/checkout-ui-extensions/latest/components/feedback/banner) </Resources> </Substep> </Step> <Step> <CheckoutUiPreview extension="banner " /> </Step> <Deploy /> <Step> ### Test the banner settings After the extension has been deployed, app users can edit the banner settings in the [checkout editor](/docs/apps/build/checkout/test-checkout-ui-extensions#test-the-extension-in-the-checkout-editor). Test the banner settings so that you can see how they appear to app users. <Substep> #### Test the the banner settings in the checkout editor 1. In the development store where your app is installed, open the checkout editor: in the Shopify admin for the development store, navigate to **Settings** > **Checkout** > **Customize**. 1. In the checkout editor, select **Add an app block**, and then select your extension. 1. In the **App block settings** section, update the banner title, description, and status. 1. To preview your changes, do the following: 1. From your development store's storefront, add products to your cart. 1. Click **Check out**. 1. View the banner on the first page of the checkout. The banner reflects the settings that you set in the checkout editor. </Substep> </Step> </StepSection> <NextSteps> ## Tutorial complete! Nice work - what you just built could be used by Shopify merchants around the world! Keep the momentum going with these related tutorials and resources. ### Next steps <CardGrid> <LinkCard href="/docs/apps/checkout/localizing-ui-extensions"> #### Localize your extension Learn how to localize the text and number formats in your extension. </LinkCard> <LinkCard href="/docs/api/checkout-ui-extensions/latest/components"> #### Explore the checkout UI extension component reference Learn about all of the components that you can use in your checkout UI extension. </LinkCard> <LinkCard href="/docs/api/checkout-ui-extensions/latest/apis/extensiontargets"> #### Explore the checkout UI extension targets API reference Learn about the extension targets offered in the checkout. </LinkCard> </CardGrid> </NextSteps>