---
gid: a8848317-e995-4c16-9edf-6cd0df40006c
title: Build a profile inline extension
description: Learn how to build Profile inline extensions by creating a loyalty app and integrating it into the profile page.
---
import CustomerAccountUiCreate from 'app/views/partials/apps/customer-accounts/ui-extensions/create.mdx'
import CustomerAccountUiPreview from 'app/views/partials/apps/customer-accounts/ui-extensions/preview.mdx'
import UiExtensionRequirements from 'app/views/partials/apps/customer-accounts/ui-extensions/requirements.mdx'
Inline extensions render after, before, or within a piece of UI, at either [static](/docs/api/customer-account-ui-extensions/extension-targets-overview#static-extension-targets) or [block](/docs/api/customer-account-ui-extensions/extension-targets-overview#block-extension-targets) extension targets.
In this tutorial, you'll build an extension for the customer **Profile** page that displays the total points earned.
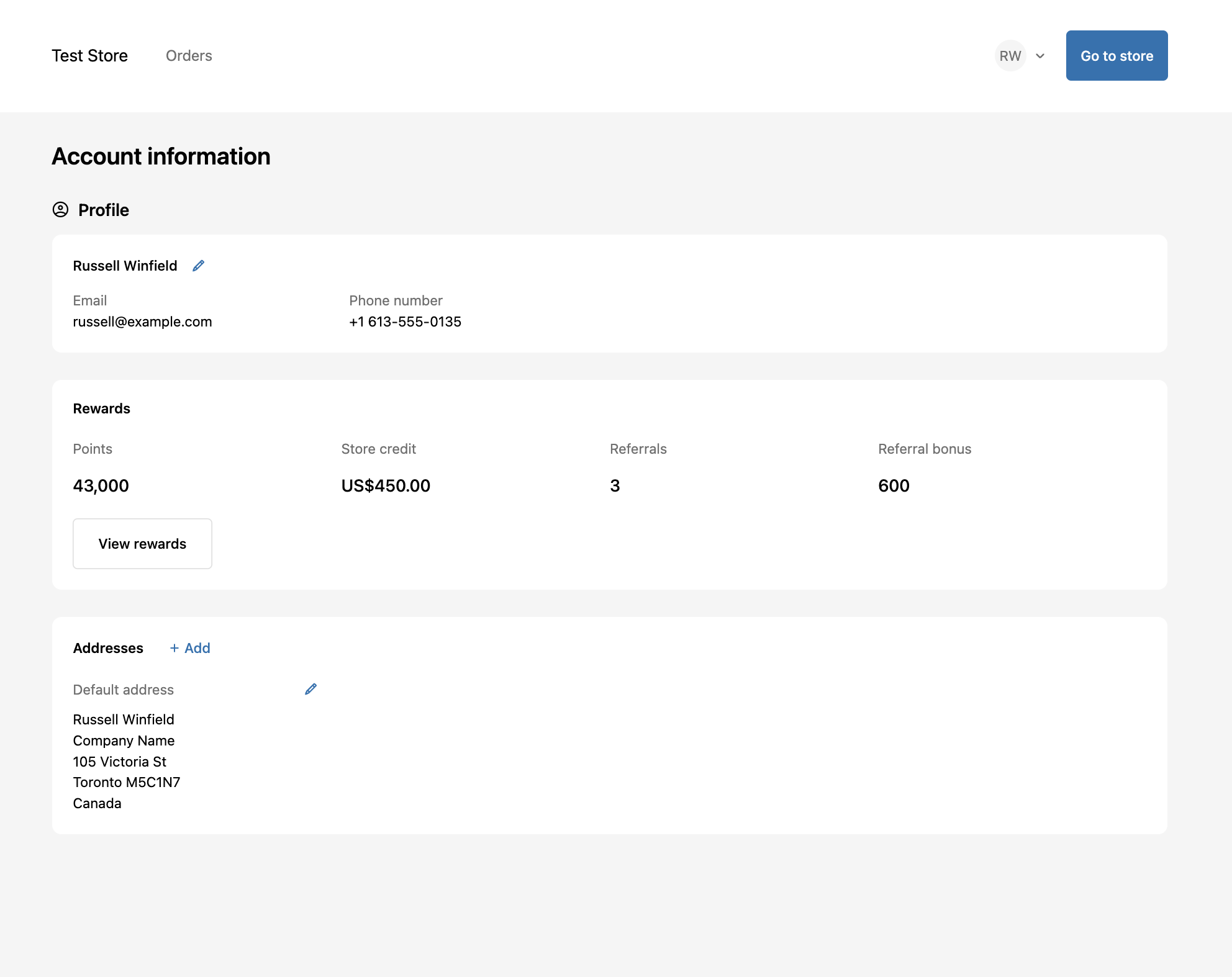
## What you'll learn
In this tutorial, you'll learn how to do the following tasks:
- Create an extension that will be render on the **Profile** page
- Run the extension locally and test it on a development store
### Create a customer account UI extension for the profile block target
### Set up the target for your extension
Set up the target for your customer account UI extension. [Targets](/docs/api/customer-account-ui-extensions/latest/targets) control where your extension renders in the customer account flow.
The example code uses the following target:
`customer-account.profile.block.render`
In your extension's configuration file, for the `customer-account.profile.block.render` target create an `[[extensions.targeting]]` section with the following information:
**`target`**: An identifier that specifies where you're injecting code into Shopify.
**`module`**: The path to the file that contains the extension code.
Create a file in your extension's `src` directory for the target. In this example, you'll create a file for the order status block extension. Make sure that the name of the files match the `module` paths [that you specified](#reference-the-targets-in-your-configuration-file).
---
[shopify.extension.toml](/docs/apps/build/app-extensions/configure-app-extensions) is the configuration file for your extension.
Whenever you edit your extension configuration file, you need to restart your server for the changes to take effect.
### Build the profile block extension
#### Build the UI
Add a card to the customer account page that displays the customer's loyalty program information, using the `Card` component and several Checkout UI extension components.
In this example, the rewards information is hardcoded. In a production-ready application, you'd retrieve this information by making an API call to your server, or to the [Customer Account API](/docs/api/customer) if you're storing loyalty and rewards data in metafields.
While this is outside of the scope of this tutorial, the example also adds a **View rewards** button, which you could link to a full-page extension that displays the customer's rewards information in more detail.
---
Checkout UI extensions are limited to specific UI components exposed by the platform [for security reasons](/docs/api/checkout-ui-extensions#security). Checkout UI components allow you to create a UI that feels seamless within the checkout experience, and that inherits a merchant's brand settings.
[Card](/docs/api/customer-account-ui-extensions/latest/components/Card)
[BlockStack](/docs/api/checkout-ui-extensions/latest/components/structure/BlockStack)
[InlineLayout](/docs/api/checkout-ui-extensions/latest/components/structure/InlineLayout)
[Heading](/docs/api/checkout-ui-extensions/latest/components/titles-and-text/Heading)
[TextBlock](/docs/api/checkout-ui-extensions/latest/components/titles-and-text/TextBlock)
[Button](/docs/api/checkout-ui-extensions/latest/components/actions/button)
### Preview the extension
Preview your extension to make sure that it works as expected.
Navigate to the **Profile** page of a customer account to see your extension in action.
___
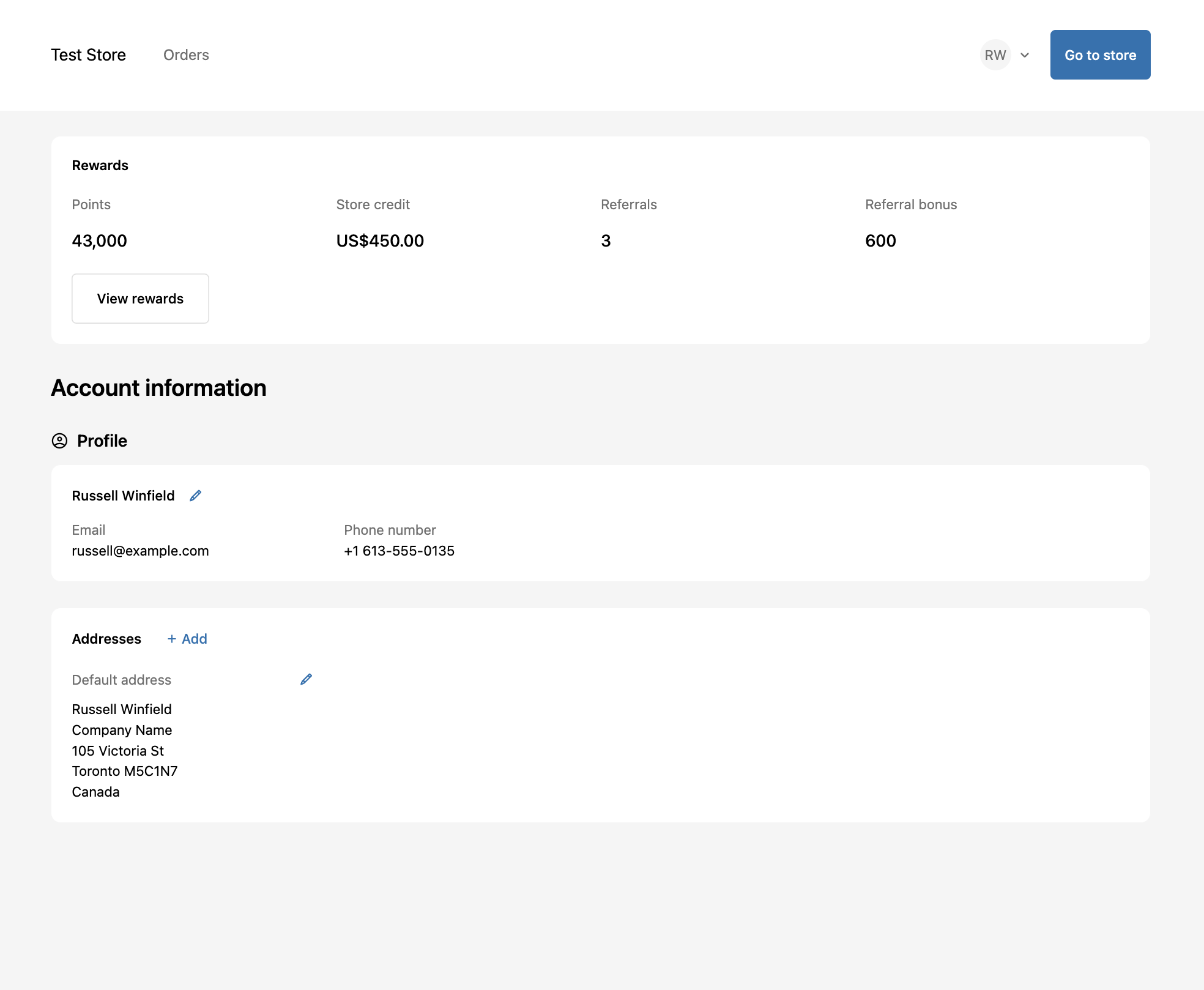
In the **Profile** page, your extension first displays at the top of the page, between the navigation header and the page title. By default, block extensions are placed in the first available placement reference.
Visually this extension should not be rendered before the heading of the page, and it should instead render in the next placement reference on the page. In a production-ready situation, merchants move this extension to the right location using the checkout editor. To test this, you can add the `?placement-reference=PROFILE1` query parameter to the URL of the **Profile** page. This moves the extension the next placement reference on the page.
Learn more about [testing UI extensions](/docs/apps/build/customer-accounts/test) in different placement references.
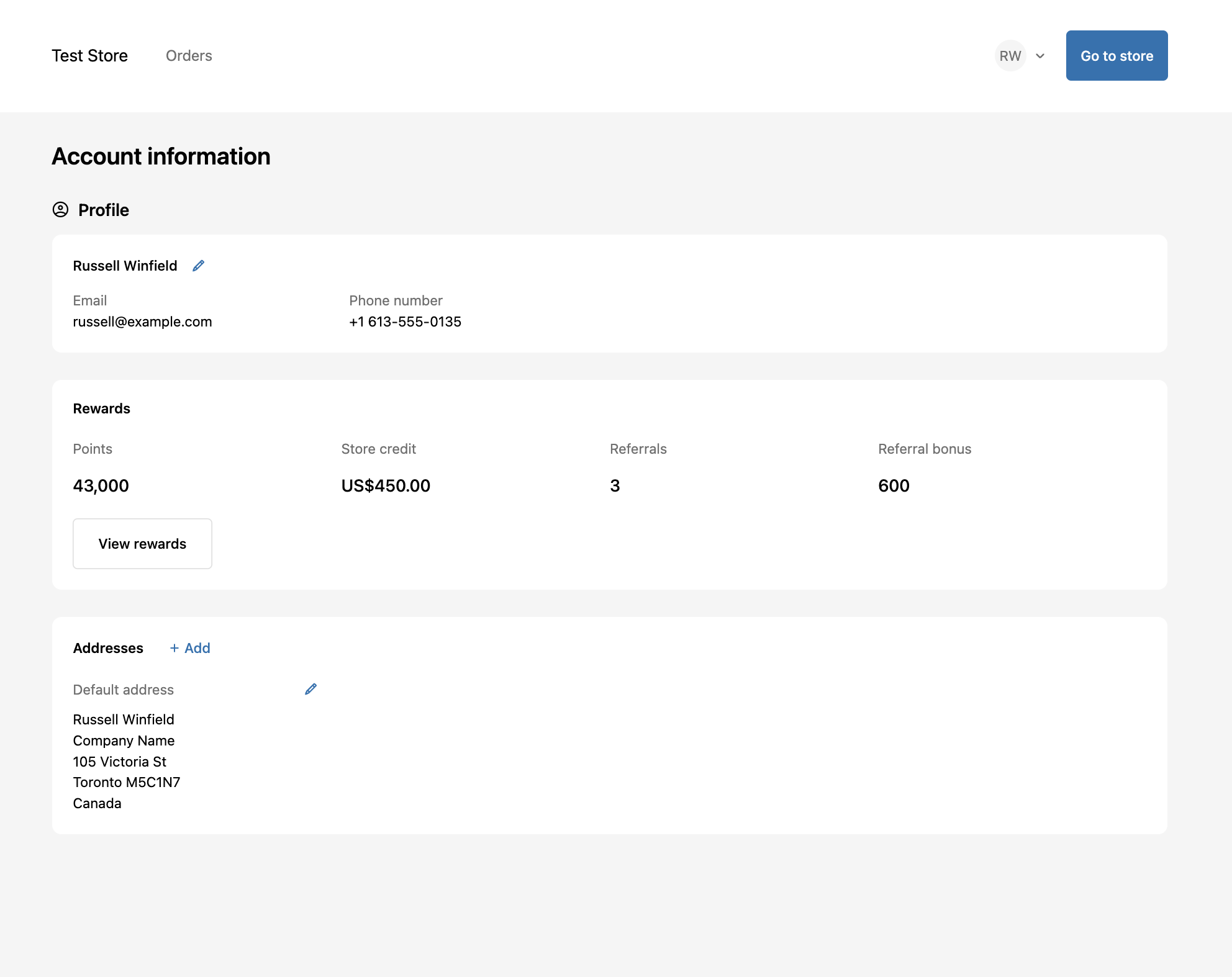
## Tutorial complete!
Nice work - what you just built could be used by Shopify merchants around the world! Keep the momentum going with these related tutorials and resources.
### Next Steps
#### Extension placement
Explore extension placement options and make informed decisions on where to position them.
#### Localize your extension
Learn about localizing your customer account UI extensions for international merchants and customers.
#### Extension targets
Learn about the extension targets offered for customer accounts.
#### UX guidelines
Follow our UX guidelines for customer accounts to ensure a consistent and satisfying user experience.
#### Customer account components
Learn about the components you can use to build customer account UI extensions.
#### Checkout components
Learn about the checkout components you can use to build customer account UI extensions.