--- gid: 2c6872d8-1f0e-4356-9784-1eed18c8dda4 title: Add a survey to Thank you and Order status pages description: Learn how to build a post-checkout survey that appears on Thank you and Order status pages using checkout UI extensions. --- import Deploy from 'app/views/partials/extensions/deploy.mdx' import CheckoutUiRequirements from 'app/views/partials/apps/checkout/ui-extensions/requirements.mdx' import CheckoutUiCreate from 'app/views/partials/apps/checkout/ui-extensions/create.mdx' import CheckoutUiReference from 'app/views/partials/apps/checkout/ui-extensions/reference.mdx' import CheckoutUiPreview from 'app/views/partials/apps/checkout/ui-extensions/preview.mdx' import CheckoutUiTroubleshooting from 'app/views/partials/apps/checkout/ui-extensions/troubleshooting.mdx' <Repo extension="react" href="https://github.com/Shopify/example-checkout--order-status--react" /> <Picker name="extension"> <PickerOption name="react" /> </Picker> <Overview> The **Thank you** and **Order status** pages are shown to customers at the end of checkout, and can be customized using checkout UI extensions. In this tutorial, you'll create a single extension that displays two different surveys depending where an app user places the extension in the checkout editor. You'll use both the `purchase.thank-you.block.render` and `customer-account.order-status.block.render` extension targets to build two surveys: - A survey that asks the customer how they heard about the store. - A survey that asks how the customer is enjoying their purchase after the order has been delivered. Follow along with this tutorial to build a sample app, or clone the completed sample app. You can use this tutorial as an example to build other customizations, such as adding a form that customers can submit to subscribe to a newsletter. 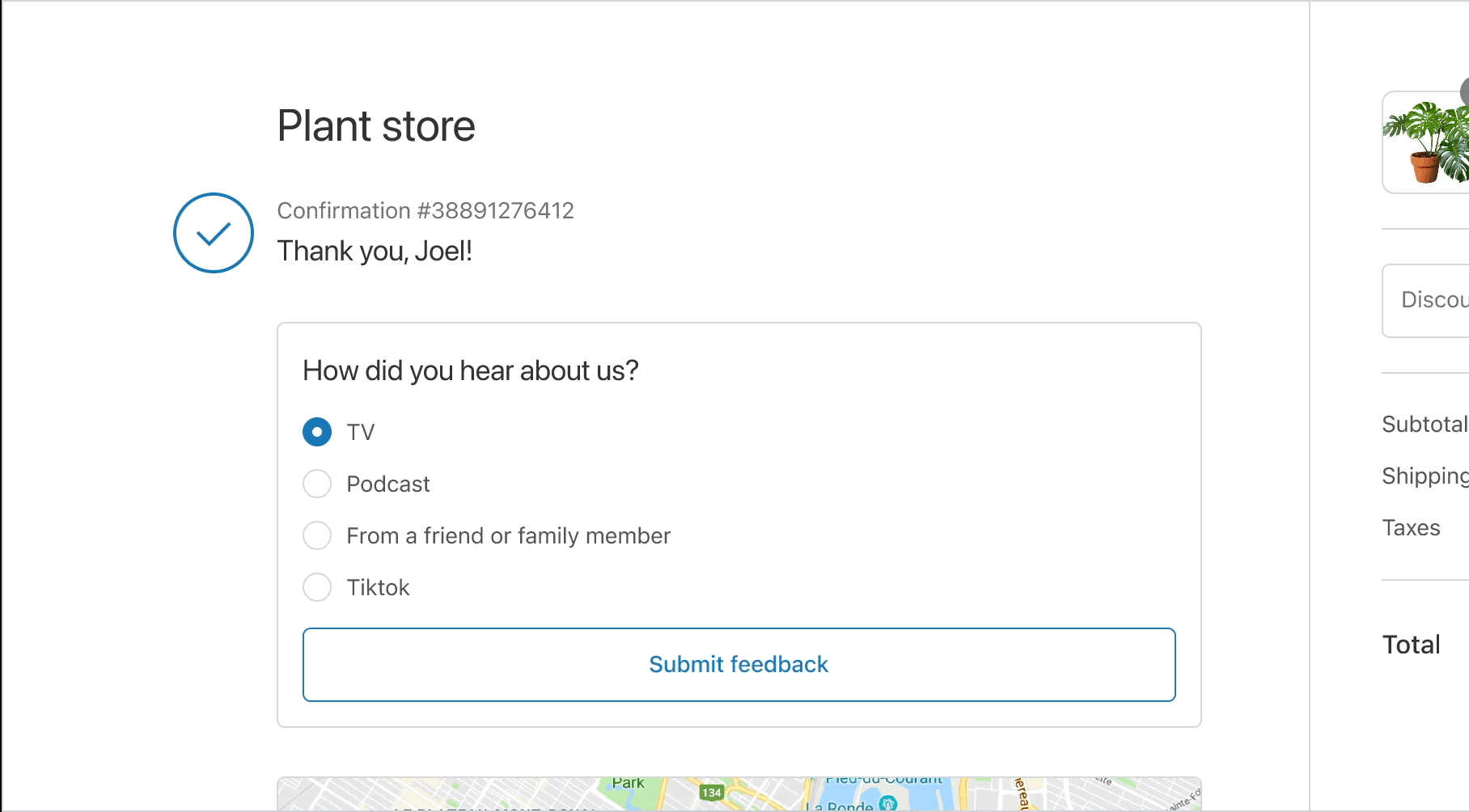 ## What you'll learn In this tutorial, you'll learn how to do the following: - Create a checkout UI extension that renders on the **Thank you** and **Order status** pages. - Use multiple extension targets to display different interfaces to the customer. - Set up the UI components for the post-checkout survey. - Run the extension locally and test it on a development store. - Deploy your extension code to Shopify. </Overview> <Requirements> <CheckoutUiRequirements /> </Requirements> <StepSection> <Step> ### Create a Checkout UI extension To create a checkout UI extension, you'll use Shopify CLI, which generates starter code for building your extension. <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--order-status--react/blob/main/extensions/order-status-thank-you/src/Checkout.jsx" /> <CheckoutUiCreate /> </Substep> </Step> <Step> ### Set up a target for your extension Set up a target for your checkout UI extension. [Targets](/docs/api/checkout-extensions/checkout#extension-targets) control where your extension renders in the checkout flow. <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--order-status--react/blob/main/extensions/order-status-thank-you/src/Checkout.jsx" tag="order-status.extension-point"/> #### Export the targets from your script file In your `Checkout.jsx` file, set the entrypoints for the checkout extension, and then export them so they can be referenced in your configuration. For each target that you want to use, create a `reactExtension` function that references your target, and export it using a named export. --- This example code uses the `purchase.thank-you.block.render` and `customer-account.order-status.block.render` targets. Adding two targets enables you to display different components for each target. Targets prefixed by `purchase.thank-you` render only on the **Thank you** page. Because the **Thank you** page is shown at the end of every checkout, you can ensure that the survey is seen by the majority of customers. When a customer visits the **Thank you** page, the order hasn't been created or delivered yet. To enable customers to review the product, you'll use a `customer-account.order-status` target to add content to the **Order status** page. The **Order status** page is shown to customers when they revisit their purchase. <Resources> [purchase.thank-you.block.render](/docs/api/checkout-ui-extensions/latest/targets/block/purchase-thank-you-block-render) [customer-account.order-status.block.render](/docs/api/checkout-ui-extensions/latest/targets/block/customer-account-order-status-block-render) </Resources> </Substep> <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--order-status--react/blob/main/extensions/order-status-thank-you/shopify.extension.toml" tag="order-status.cofig-extension"/> <CheckoutUiReference /> </Substep> </Step> <Step> ### Build the survey UI Build a user interface using components from the [checkout UI extensions](/docs/api/checkout-ui-extensions/latest/components) component library. <Substep> <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--order-status--react/blob/main/extensions/order-status-thank-you/src/Checkout.jsx" tag="order-status.survey-component"/> #### Create a survey component Create a function to set up checkout UI components that the two surveys share, including a button to submit feedback and a message that appears after the feedback is submitted. --- Checkout UI extensions are limited to specific UI components exposed by the platform [for security reasons](/docs/api/checkout-ui-extensions#security). Checkout UI components allow you to create a UI that feels seamless within the checkout experience, and that inherits a merchant's brand settings. <Resources> [View](/docs/api/checkout-ui-extensions/latest/components/structure/view) [BlockStack](/docs/api/checkout-ui-extensions/latest/components/structure/blockstack) [Heading](/docs/api/checkout-ui-extensions/latest/components/titles-and-text/heading) [Text](/docs/api/checkout-ui-extensions/latest/components/titles-and-text/text) [Button](/docs/api/checkout-ui-extensions/latest/components/actions/button) </Resources> </Substep> <Substep> #### Create an attribution survey <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--order-status--react/blob/main/extensions/order-status-thank-you/src/Checkout.jsx" tag="order-status.attribution-survey"/> Use the `Survey` component that you created, along with checkout UI components, to build an attribution survey interface. This UI includes a `ChoiceList` to specify how the customer heard about the store. --- This sample code simulates storing the survey response by logging to your console. In a production-ready application, you should store the survey response in a database, or in [metafields](/docs/apps/custom-data/). This sample code also uses hard-coded survey questions and options. In a production-ready application, you should allow app users to customize the survey questions and answers with [checkout UI extension settings](/docs/api/checkout-ui-extensions/latest/configuration#settings-definition). Learn more about the [UX guidelines](/docs/apps/checkout/thank-you-order-status/ux-guidelines) for these pages. <Resources> [ChoiceList](/docs/api/checkout-ui-extensions/latest/components/forms/choicelist) [Choice](/docs/api/checkout-ui-extensions/latest/components/forms/choice) [BlockStack](/docs/api/checkout-ui-extensions/latest/components/structure/blockstack) </Resources> </Substep> <Substep> #### Create a product review survey <CodeRef extension="react" href="https://github.com/Shopify/example-checkout--order-status--react/blob/main/extensions/order-status-thank-you/src/Checkout.jsx" tag="order-status.product-review"/> Use the `Survey` component that you created, along with checkout UI components, to build a product review survey interface. This UI includes a `ChoiceList` to specify how the customer is enjoying their purchase. --- <Resources> [ChoiceList](/docs/api/checkout-ui-extensions/latest/components/forms/choicelist) [Choice](/docs/api/checkout-ui-extensions/latest/components/forms/choice) [BlockStack](/docs/api/checkout-ui-extensions/latest/components/structure/blockstack) </Resources> </Substep> </Step> <Step> <CheckoutUiPreview extension="Order status page "/> <Substep> #### Test the attribution survey 1. Complete the checkout by [placing a test order](https://help.shopify.com/manual/checkout-settings/test-orders). When you visit the **Thank you** page, the attribution survey displays. 1. Refresh the page to access the product review survey on the **Order status** page. 1. Optional: To preview the dynamic extension in a different supported location, add the [placement-reference URL](/docs/apps/build/checkout/test-checkout-ui-extensions#dynamic-extension-points) query parameter. --- To remain on the **Thank you** page through a page refresh, append the `?prevent_order_redirect=true` parameter to the URL. This parameter persists in the local storage of your browser and prevents redirection to the **Order status** page. To re-enable redirection, either clear your local storage or add the `?prevent_order_redirect=false` parameter to the URL and reload the page. <CheckoutUiTroubleshooting /> </Substep> </Step> <Deploy /> </StepSection> <NextSteps> ## Tutorial complete! Nice work - what you just built could be used by Shopify merchants around the world! Keep the momentum going with these related tutorials and resources. ### Next steps <CardGrid> <LinkCard href="/docs/apps/checkout/thank-you-order-status/ux-guidelines"> #### Review order status and **Thank you** page UX guidelines Optimize your user experience by following our UX guidelines. </LinkCard> <LinkCard href="/docs/apps/checkout/localizing-ui-extensions"> #### Localize your extension Learn how to localize the text and number formats in your extension. </LinkCard> <LinkCard href="/docs/api/checkout-ui-extensions/latest/components"> #### Explore the checkout UI extension component reference Learn about all of the components that you can use in your checkout UI extension. </LinkCard> <LinkCard href="/docs/api/checkout-ui-extensions/latest/apis/extensiontargets"> #### Explore the checkout UI extension targets API reference Learn about the extension targets offered in the checkout. </LinkCard> </CardGrid> </NextSteps>