Build a customer segment action extension
A customer segment action extension is an action extension that is displayed in the Use segment modal.
This guide is the second part in a two-part tutorial series on how to build a feature using customer segment template extensions and customer segment action extensions in the Use segment modal. The first guide in the series is not required to complete the second part of the tutorial.
This guide demonstrates how to build a customer segment action extension that enables users to perform actions on a target customer segment. This action will appear under the customer segment details page, in the Use segment modal.
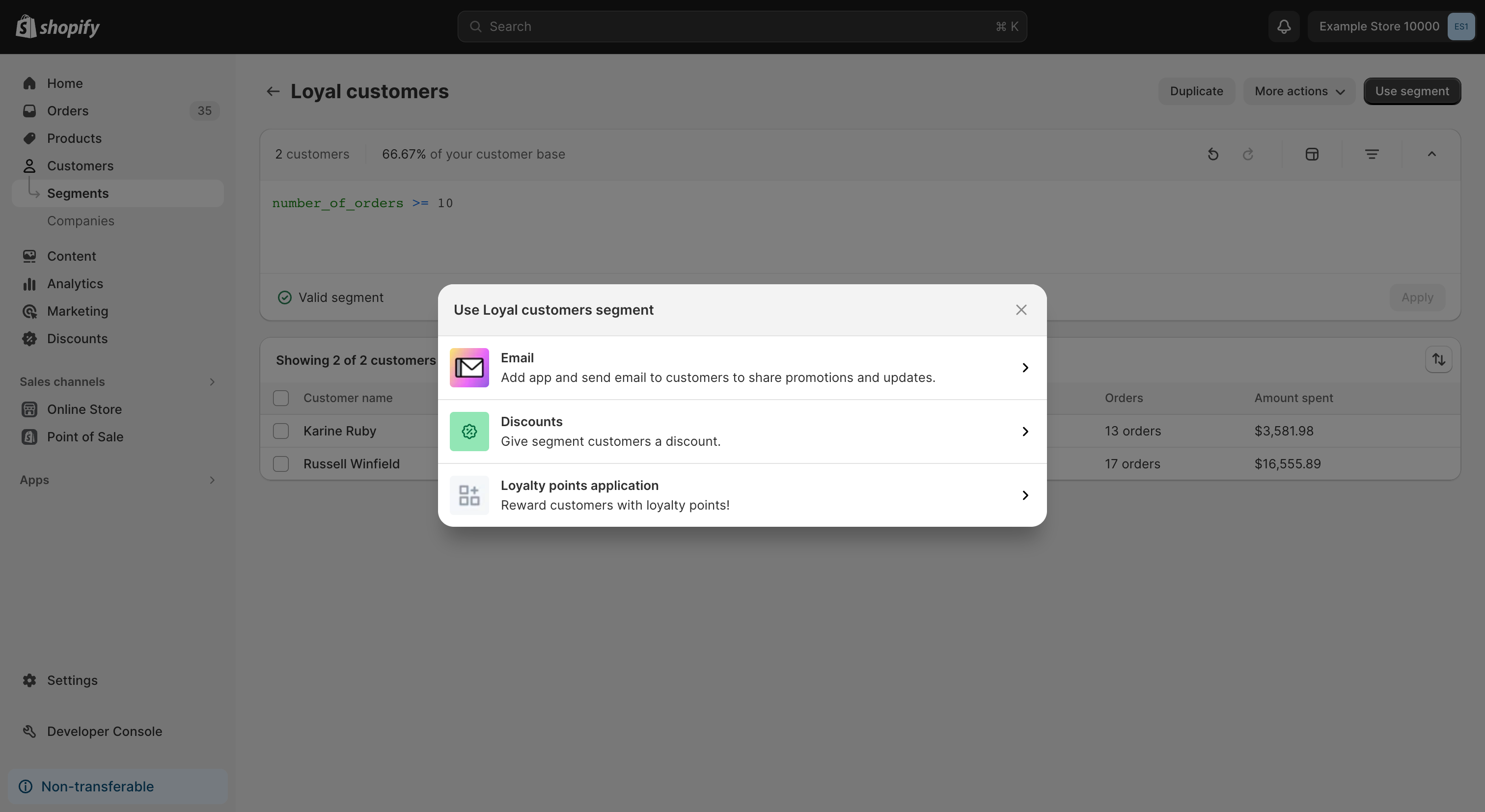
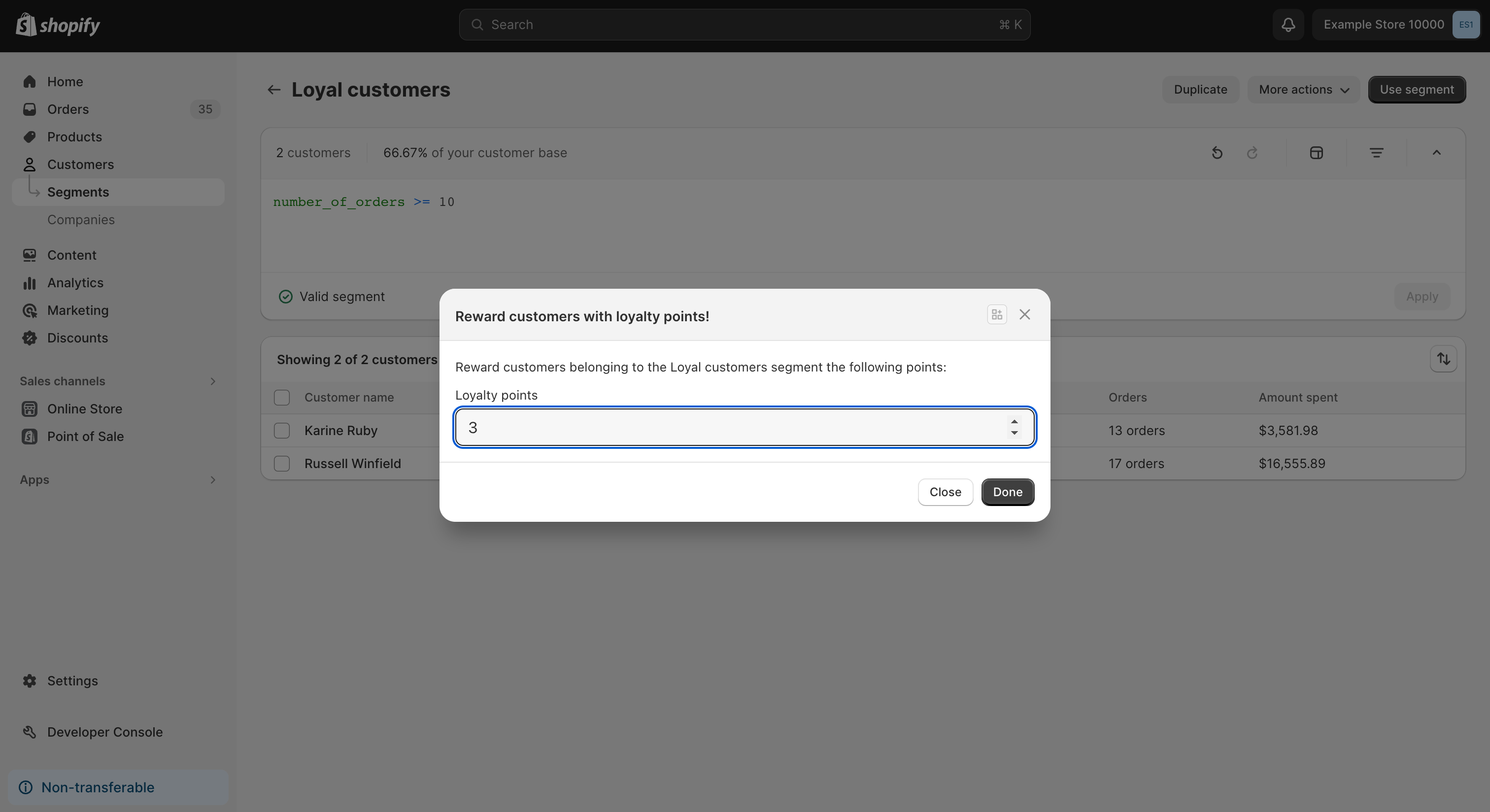
What you'll learn
Anchor link to section titled "What you'll learn"In this tutorial, you'll learn how to do the following tasks:
Create a customer segment action extension that displays on the customer segment details page or the customer segments index page.
Fetch information to populate the extensions's initial state.
Configure the extension to gather input from users.
Update the data using GraphQL based on user input.
Run the extension locally and test it on a development store.
Requirements
Anchor link to section titled "Requirements"You've created a Partner account and a development store.
Your app is using Shopify CLI 3.48 or higher.
Your app can make authenticated requests to the GraphQL Admin API.
Your app has the
read_customers
andwrite_customers
access scopes. Learn how to configure your access scopes using Shopify CLI.
Step 1: Create a new extension
Anchor link to section titled "Step 1: Create a new extension"To create your customer segment action extension, you can use Shopify CLI to generate a starter extension.
Navigate to your app directory:
Run the following command to create a new admin action extension:
The command creates a new extension template in your app's
extensions
directory with the following structure:
Step 2: Build the extension's UI
Anchor link to section titled "Step 2: Build the extension's UI"Complete the following steps to build the extension's UI.
Review the configuration
Anchor link to section titled "Review the configuration"The extension's .toml
file stores the extension's static configuration. To have the action appear in the Use segment modal, validate that the target
is set to admin.customer-segment-details.action.render
. For a full list of targets and the locations where they display in the Shopify admin, refer to the admin extension configuration reference.
To update the display name when users select the action from the menu, in locale files edit the corresponding name
value.
Create the UI
Anchor link to section titled "Create the UI"Admin UI extensions are rendered using Remote UI, which is a fast and secure remote-rendering framework. Because Shopify renders the UI remotely, components used in the extensions must comply with a contract in the Shopify host. We provide these components through the UI Extensions library. For a list of all the available components and their props, refer to the admin UI extension component reference.
You can view the source of your extension in the src/ActionExtension.jsx
file. This file defines a functional React component that's exported to run at the extension's target. You can create the extension's body by importing and using components from the @shopify/ui-extensions-react/admin
package.
To build your action's UX, add the following code to src/ActionExtension.jsx
, and the locales to locales/en.default.json
.
Step 3: Build the extension logic and connect to the GraphQL Admin API
Anchor link to section titled "Step 3: Build the extension logic and connect to the GraphQL Admin API"After defining the UI of the extension, you can complete the experience by building the logic to control it using standard React tooling.
When you're building extensions, you don't need proxy calls to the GraphQL Admin API through your app's backend. Instead, your extension can use direct API access to create requests directly using fetch
. This helps extensions be more performant and responsive for users. This guide includes a utility file for GraphQL queries. Add the contents below to a new file at ./src/utils.js
.
Your app can also get data from the extension APIs, which includes data on the current resource from the data
API.
Step 4: Test the extension
Anchor link to section titled "Step 4: Test the extension"After you've built the extension, you can test it by completing the following steps.
Navigate to your app directory:
To build and preview your app, either start or restart your server with the following command:
Press
p
to open the developer console.In the developer console page, click on the preview link for the loyalty points action extension.
The customer segment details page opens. If you don't have a customer segment in your store, then you need to create one.
To launch your extension, click the Use segment button and select your extension.
After you've entered a number of loyalty points, click Done.
Validate that the loyalty points metafield has properly been updated by navigating to one of the customer profiles that belongs to your segment. Click Show all in the Metafields section. The loyalty points metafield should display the correct value.
- Learn about the surfaces in the Shopify admin where you can create admin extensions.
- Learn about the full set of available components for writing admin UI extensions.