Localize a checkout UI extension
In this tutorial, you'll use JavaScript API functions to localize an extension that displays a customer's loyalty point balance. You'll localize the extension text, the number format of the loyalty points balance, and the monetary value of the points. You'll also provide translations for singular and plural values. You can use what you learn here to localize other extensions.
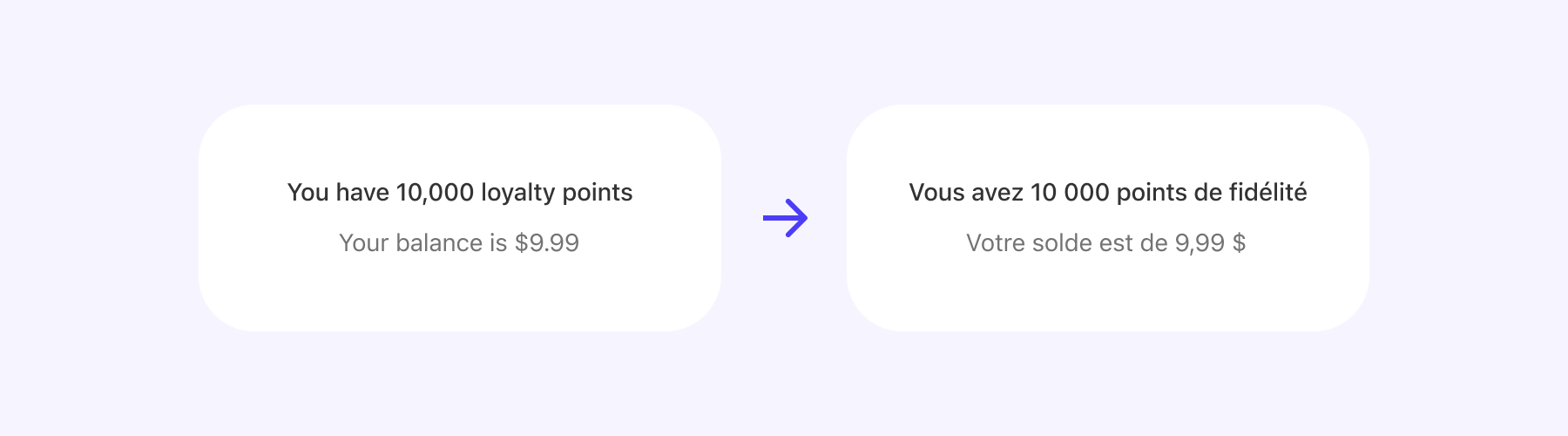
What you'll learn
Anchor link to section titled "What you'll learn"In this tutorial, you'll learn how to do the following tasks:
Create a checkout UI extension that renders some text in the checkout flow with some basic localization.
Run the extension locally and test it on a development store.
Define translation data and localize the following elements:
- Numbers using a
formatNumber
function similar to theIntl
object - Currency using a
formatCurrency
Intl
object - Singular and plural values
- Numbers using a
Deploy your extension code to Shopify.
Requirements
Anchor link to section titled "Requirements"You've created a Partner account.
You've created a new development store with the following:
You're familiar with how localization works for checkout UI extensions.
You've added and published a second language to your development store.
You've activated the language in your development store's primary market.
Sample code
Anchor link to section titled "Sample code"You can copy and paste the following code into your index
file and add a few example values to get the extension to render in the browser.
The rest of the tutorial walks through this sample code step-by-step.
Step 1: Create a UI extension
Anchor link to section titled "Step 1: Create a UI extension"To create a checkout UI extension, you can use Shopify CLI, which generates starter code for building your extension and automates common development tasks.
Navigate to your app directory:
Run the following command to create a new checkout UI extension:
Select a language for your extension. You can choose from TypeScript, JavaScript, TypeScript React, or JavaScript React.
You should now have a new extension directory in your app's directory. The extension directory includes the extension script at
src/index.{file-extension}
. The following is an example directory structure:Start your development server to build and preview your app:
To learn about the processes that are executed when you run
dev
, refer to the Shopify CLI command reference.Press
p
to open the developer console. In the developer console page, click on the preview link for your extension.
Step 2: Define translations
Anchor link to section titled "Step 2: Define translations"To define translations, you'll adjust the [locale].json
files in the extensions/<name-of-checkout-ui-extension>/locales
folder within your app.
Set the default locale
Anchor link to section titled "Set the default locale"Your default locale specifies which locale Shopify should use when no other appropriate locale can be matched. In this example, English (en
) is already the default locale. However, you can set any locale to be your default locale.
To change your default locale, go to the locales
folder and change the [locale].json
filename to [locale].default.json
.
Add translation strings
Anchor link to section titled "Add translation strings"In this step, you'll add translations for different plural forms. You'll set translations for the many
, one
and other
plural rules needed for French (fr
), but you can specify any pluralization key that Intl.PluralRules.select()
supports and that's appropriate for the locale.
In subsequent steps, you'll define balance
and points
using a placeholder.
In
en.default.json
, add the following code:In the
fr.json
file, add the translated content:
Step 3: Localize the currency
Anchor link to section titled "Step 3: Localize the currency"Now that you've defined translations, you'll learn how to localize currency.
You'll add the formatCurrency
function provided by i18n
. The function wraps the standard Intl
object.
Depending on the current locale, 9.99
will now resolve to the following localized currency formats:
en
:$9.99
fr
:9,99
Step 4: Localize numbers
Anchor link to section titled "Step 4: Localize numbers"In this step, you'll learn how to resolve localized numbers.
You'll localize number formatting using the formatNumber
function provided by i18n
. The function wraps the standard Intl
object.
Depending on the current locale, 10000
will resolve to one of the following localized number formats:
en
:10,000
fr
:10 000
Step 5: Translate the balance remaining message
Anchor link to section titled "Step 5: Translate the balance remaining message"In this step, you'll learn how to translate the balance remaining message using a placeholder.
You'll use a placeholder for formattedBalance
. You'll also call the translate
function, which sends the formattedBalance
variable so that it can be used in the translation string.
Step 6: Translate the loyalty points message with plural values
Anchor link to section titled "Step 6: Translate the loyalty points message with plural values"In this step, you'll learn how to translate the loyaltyPoints
message, which supports pluralization.
You'll use the translate
function to pass in the count
of how many points are available. You'll use formattedPoints
to render the points in the current locale.
The checkout UI extension should now render localized checkout content for en
and fr
:
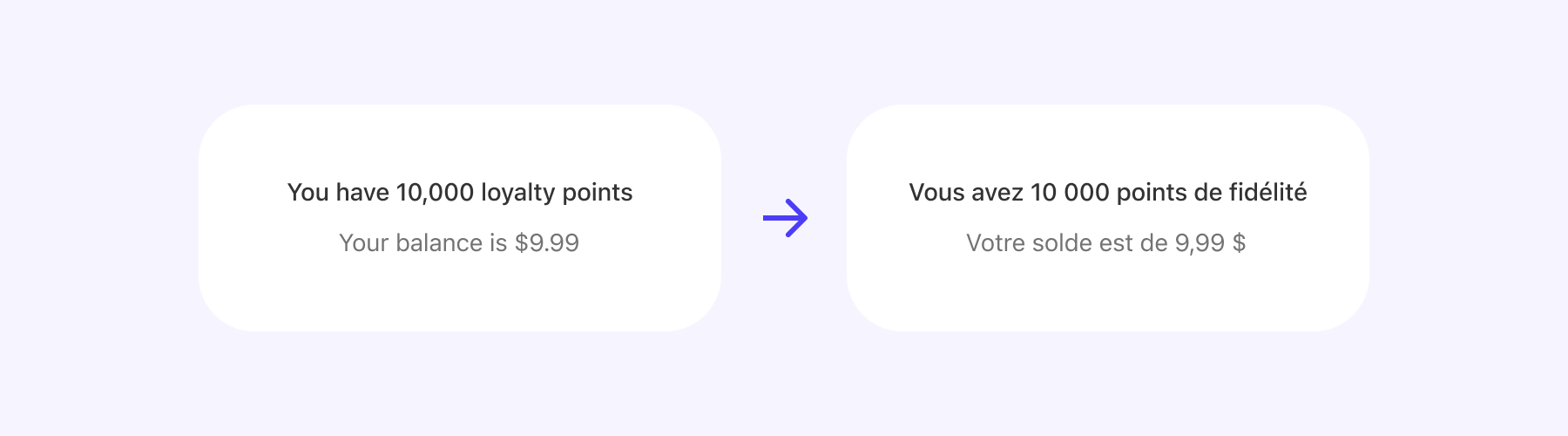
To test the extension, preview the language from your development store admin.
Step 7: Deploy the UI extension
Anchor link to section titled "Step 7: Deploy the UI extension"When you're ready to release your changes to users, you can create and release an app version. An app version is a snapshot of your app configuration and all extensions.
You can have up to 50 checkout UI extensions in an app version.
- Navigate to your app directory.
Run the following command.
Optionally, you can provide a name or message for the version using the
--version
and--message
flags.
Releasing an app version replaces the current active version that's served to stores that have your app installed. It might take several minutes for app users to be upgraded to the new version.
Troubleshooting
Anchor link to section titled "Troubleshooting"This section describes how to solve some potential errors when you run dev
for an app that contains a checkout UI extension.
Property token error
Anchor link to section titled "Property token error"If you receive the error ShopifyCLI:AdminAPI requires the property token to be set
, then you'll need to use the --checkout-cart-url
flag to direct Shopify CLI to open a checkout session for you.
Missing checkout link
Anchor link to section titled "Missing checkout link"If you don't receive the test checkout URL when you run dev
, then verify the following:
You have a development store populated with products.
You're logged in to the correct Partners organization and development store. To verify, check your app info using the following command:
Otherwise, you can manually create a checkout with the following steps:
From your development store's storefront, add some products to your cart.
From the cart, click Checkout.
From directory of the app that contains your extension, run
dev
to preview your app:On the checkout page for your store, change the URL by appending the
?dev=https://{tunnel_url}/extensions
query string and reload the page. Thetunnel_url
parameter allows your app to be accessed using a unique HTTPS URL.You should now see a rendered order note that corresponds to the code in your project template.
- Use JavaScript APIs to access translations for localizing checkout UI extensions.