Connect wallet
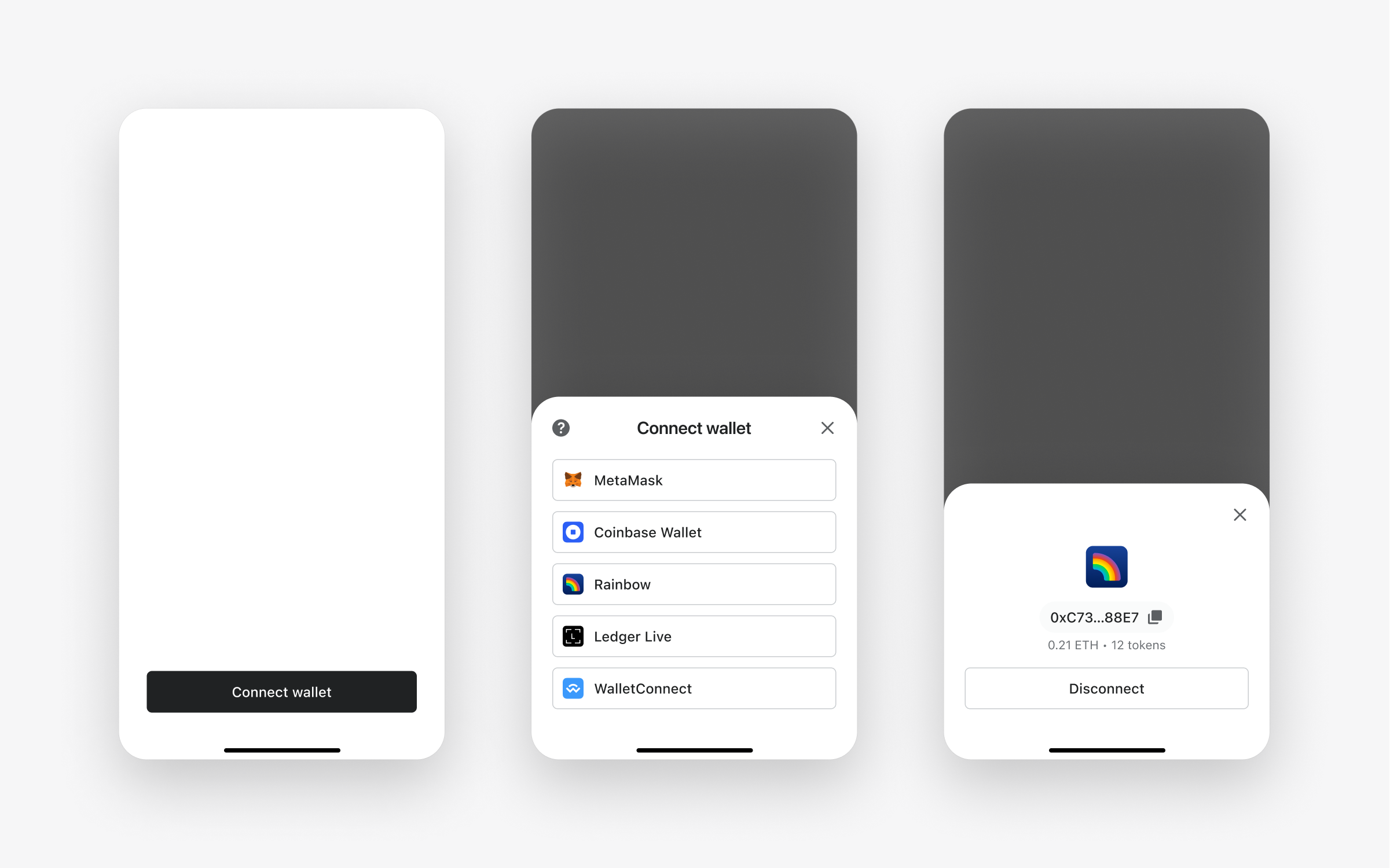
The @shopify/connect-wallet
package provides a standard way of connecting wallets and signing messages on Shopify storefronts.
Get started
Anchor link to section titled "Get started"To get started with using @shopify/connect-wallet
you need to follow these steps:
- Installation
- Client configuration
- App provider setup
- Adding the
<ConnectButton />
component to your app - Custom connect button
Installation
Anchor link to section titled "Installation"
Client configuration
Anchor link to section titled "Client configuration"Retrieve the
projectId
for your WalletConnect app by opening the WalletConnect dashboard and navigating to your project.In your project's root folder, create a new file called
connect-wallet-config.js
.Add the following code:
App provider setup
Anchor link to section titled "App provider setup"Let's begin using the configured client and chains. In your app's entry point, (either index.tsx
, _app.tsx
, or your framework's entry point), wrap the rendered component with both <WagmiConfig />
and the <ConnectWalletProvider />
as follows.
Adding the ConnectButton
component to your app
Anchor link to section titled "Adding the ConnectButton component to your app"This example shows a pseudo code version of including the component in your navigation header, but you're welcome to place the component where you prefer.
Custom connect button
Anchor link to section titled "Custom connect button"Don't like our connect button? All good! You can replace our button with your own and use the openModal
function returned from the useConnectionModal
hook.
Connectors allow users to connect to their preferred wallet app.
buildConnectors
Anchor link to section titled "buildConnectors"We also support adding custom connectors and editing the list of supported wallets for users to connect with. In the connect-wallet-config.ts
file you created during initial configuration, you can pass additional values to the buildConnectors
function to do so.
Name | Description | Type | Required | Default Value |
---|---|---|---|---|
customConnectors |
A list of the custom connectors supported by your app. The custom connector types currently supported are InjectedConnector and WalletConnectConnector from wagmi. |
CustomConnector[] |
false |
undefined |
includeDefaults |
A boolean value indicating whether you choose to include the list of default connectors. If customConnectors are provided, the default connectors will be appended to the end of the list of custom connectors. |
boolean |
false |
true |
excludedConnectors |
A list of default connector id s to be excluded. View default connectors A value for excludedConnectors can only be provided when includeDefaults is true . |
string | undefined |
false |
undefined |
Return value
Anchor link to section titled "Return value"Name | Description | Type |
---|---|---|
connectors |
A list of the connectors supported by your app. Used by the ConnectWalletProvider . |
Connector[] |
wagmiConnectors |
A list of the wagmi connectors supported by your app. Used by the createClient function during client configuration. |
@wagmi/core/Connector |
Default Connectors
Anchor link to section titled "Default Connectors"Name | ID |
---|---|
MetaMask | metaMask |
Coinbase | coinbaseWallet |
Ledger Live | ledger Ledger Live connections use WalletConnect as oppposed to Ledger Connect Kit. We plan to support Ledger Connect Kit as the preferred method for connecting your Ledger Wallet in the future. Read more about Ledger Connect Kit and the Ledger Extension |
Rainbow | rainbow |
WalletConnect | walletConnect |
The following is an example of adding a custom WalletConnectConnector
and removing rainbow from the list:
You can use any of the supported chains from wagmi for @shopify/connect-wallet
. In the connect-wallet-config.ts
file you created during initial configuration, change the value provided to configureChains
like this:
For more information about configuring providers, refer to wagmi documentation.
When initializing the package, we strongly recommended including an Alchemy or Infura provider to reduce the risk of rate limiting. If your app is rate limited your customers can't connect their wallets.
For more information, refer to wagmi's configuring chains documentation.
There are three components exported from the package for your use:
ConnectButton
Anchor link to section titled "ConnectButton"An out of the box component that controls the connection and disconnection flow for wallet connection.
ConnectorIcon
Anchor link to section titled "ConnectorIcon"A component that displays the icon for a given connector ID. This component is useful for developing a custom connect button.
Name | Description | Type | Required | Default Value |
---|---|---|---|---|
id |
The id of the wallet whose icon you would like to display. |
"coinbase" | "ledger" | "metaMask" | "rainbow" | "walletConnect" |
false |
"unknown" |
size |
A string value representing the size of the icon you would like to render. | "xs" | "sm" | "md" | "lg" | "xl" Size values: "xs" -> 20px"sm" -> 24px"md" -> 40px"lg" -> 48px"xl" -> 76px |
true |
undefined |
ConnectWalletProvider
Anchor link to section titled "ConnectWalletProvider"The core component for ensuring your connect wallet integration works as expected. This component must wrap your app and be placed within a WagmiConfig
.
Name | Description | Type | Required | Default Value |
---|---|---|---|---|
chains |
A list of the chains supported by your app. | Chain[] |
true |
undefined |
connectors |
A list of the connectors supported by your app. | Connector[] |
true |
undefined |
enableDelegateCash |
A boolean value indicating whether you choose to support delegate.cash wallets. | boolean |
false |
true |
orderAttributionMode |
Dictates how automatic order attribution functions. | OrderAttributionMode |
false |
"required" |
requireSignature |
A boolean value indicating whether you choose to require signatures when a wallet connects. | boolean |
false |
true |
statementGenerator |
Any promise-based function that returns a string to provide to the message after a wallet connects. | StatementGenerator |
false |
undefined |
delegate.cash
Anchor link to section titled "delegate.cash"The enableDelegateCash
property dictates whether your app supports delegate wallets registered with the delegate.cash service. The service enables users to link cold wallets with hot wallets, and then the hot wallet can act on behalf of the cold wallet to connect and sign messages.
Supporting delegate.cash empowers users with valuable assets to confidently interact with your app knowing their assests are secured. Learn more by reading the delegate.cash documentation.
Order attribution mode
Anchor link to section titled "Order attribution mode"The orderAttributionMode
property dictates how automatic order attribution functions after successful wallet connection.
Order attribution is a feature provided for Shopify Online Storefronts. When enabled, a request dispatches to Shopify, adding the buyer's wallet address to a completed order with the name "Wallet Address". This allows users to associate an order with a particular wallet address.
Value | Description |
---|---|
disabled |
When order attribution is disabled , no attempt to perform order attribution is made. |
ignoreErrors |
When order attribution is ignoreErrors , any error that occurs during attribution will be logged to the console but ignored. |
required |
When order attribution is required , any errors as a result of the order attribution process will be thrown. |
Statement generator
Anchor link to section titled "Statement generator"The statementGenerator
prop allows you to customize the statement displayed in a Sign-In with Ethereum message. The function receives the address of the wallet that has connected, allowing you to expand and customize your message statements to better suit your brand.
Below is a sample which fetches a custom message from an endpoint with the wallet address as a parameter. The example endpoint then returns JSON data with message
as a value in the body. This isn't a guideline for how this must be used, but rather an example of what's possible.
There are two hooks exported from the package for your use:
useConnectionModal
Anchor link to section titled "useConnectionModal"The useConnectionModal
hook returns two functions which control the interaction state of the modal.
Return value
Anchor link to section titled "Return value"Name | Description |
---|---|
closeModal |
A function used to close the connect wallet modal. |
openModal |
A function used to open the connect wallet modal. |
useConnectWallet
Anchor link to section titled "useConnectWallet"A hook that returns information related to the active connected wallet, connection status, and more.
Name | Description | Type | Required | Default Value |
---|---|---|---|---|
onConnect |
A callback invoked on successful connection of a wallet. | ((wallet: Wallet) => void) | undefined |
false |
undefined |
onDisconnect |
A callback invoked when a wallet disconnects. This also returns the wallet that disconnected. | ((wallet?: Wallet) => void) | undefined |
false |
undefined |
Return value
Anchor link to section titled "Return value"Name | Description | Type |
---|---|---|
chains |
A collection of chains in use by the ConnectWalletProvider . |
Chain[] |
connecting |
A boolean value indicating whether a wallet is in the process of connecting. | boolean |
connectedWallets |
A collection of connected wallets. The package supports a single wallet connection at the moment, but this expands the capabilities of the package. | Wallet[] |
disconnect |
A function used to disconnect a wallet address. If an address is not provided, the activeWallet is disconnected. |
(address?: string => void) |
pendingConnector |
The connector being used to connect a new wallet. | SerializedConnector | undefined |
pendingWallet |
A wallet that is pending a signature request. Once the signature request completes, verification against the signature takes place. If valid, the wallet is added to the connectedWallets collection. |
Wallet[] | undefined |
signing |
A boolean value indicating whether a signature request is pending. | boolean |
wallet |
The current connected wallet. | Wallet |
We export the types used by the package to ensure that you can type your callback functions, custom connectors, and have a better understanding of how all of the exports tie together.
Name | Description | Type |
---|---|---|
connector |
The underlying wagmi connector used to connect a wallet. | @wagmi/core/Connector |
desktopAppLink |
A link prefix for WalletConnect desktop applications such as Ledger Live. | string | undefined |
icon |
The icon representing this wallet application. | JSX.Element | null |
id |
The identifier for the connector, such as metaMask . |
string |
marketingSite |
The marketing website for a connector. | string | undefined |
mobileAppPrefixes |
A record of strings with prefixes for opening a WalletConnect deeplink on Android and iOS devices. | Record<string, string | undefined> | undefined |
modalConnector |
A connector used for opening the default WalletConnect modal. | WalletConnectConnector | undefined |
name |
The name for the connector, such as MetaMask . |
string |
qrCodeSupported |
Whether or not connecting by QR Code is possible. | boolean |
ready |
Whether the connector has initialized or not. | boolean |
CustomConnector
Anchor link to section titled "CustomConnector"Name | Description | Type |
---|---|---|
connector |
The custom wagmi connector used to connect a wallet. | InjectedConnector | WalletConnectConnector |
desktopAppLink |
A link prefix for WalletConnect desktop applications such as Ledger Live. | string | undefined |
icon |
The icon representing this wallet application. SVGs should have a height and width property of 100% . |
JSX.Element | null |
id |
The identifier for the connector, such as metaMask . |
string |
marketingSite |
The marketing website for a connector. | string | undefined |
mobileAppPrefixes |
A record of strings with prefixes for opening a WalletConnect deeplink on Android and iOS devices. | Record<string, string | undefined> | undefined |
modalConnector |
A connector used for opening the default WalletConnect modal. | WalletConnectConnector | undefined |
name |
The name for the connector, such as MetaMask . |
string |
qrCodeSupported |
Whether or not connecting by QR Code is possible. | boolean |
SerializedConnector
Anchor link to section titled "SerializedConnector"Name | Description | Type |
---|---|---|
desktopAppLink |
A link prefix for WalletConnect desktop applications such as Ledger Live. | string | undefined |
id |
The identifier for the connector, such as metaMask . |
string |
marketingSite |
The marketing website for a connector. | string | undefined |
mobileAppPrefixes |
A record of strings with prefixes for opening a WalletConnect deeplink on Android and iOS devices. | Record<string, string | undefined> | undefined |
name |
The name for the connector, such as MetaMask . |
string |
qrCodeSupported |
Whether or not connecting by QR Code is possible. | boolean |
Name | Description | Type |
---|---|---|
address |
The public address of the connected wallet. | string |
connectedAt |
ISO datetime string in which this wallet connected. | string | undefined |
connectorId |
The id associated with how this wallet connected. |
string | undefined |
connectorName |
The connector name associated with how this wallet connected. | string | undefined |
message |
The message which the wallet signed. Will be undefined in the event that the wallet has not yet signed the message or if requireSignature is false . |
string | undefined |
signature |
The signed message from the signature transaction. Will be undefined in the event that the wallet has not yet signed the message or if requireSignature is false . |
string | undefined |
signedOn |
ISO datetime string in which the wallet passed the validation check. | string | undefined |