> Plus: > This payment method is only available to be installed by Shopify Plus plans. Redeemables payments extension enable merchants to integrate their external gift card provider as a payment method at checkout. Your payment extension enables customers to apply gift cards directly on the checkout page, without having to redirect them to an offsite page. Your payments extension will provide a [Checkout UI extension](/docs/api/checkout-ui-extensions) and [Balance Request](#step-1-fetch-balance) endpoint to enable Shopify to check the balance of gift cards during checkout. <img src="/assets/apps/payments/gift-card-payment-method-app-example.png" alt="Example gift card payment method" height="300px" /> ## Step 1: Scaffold an app > Tip: > Most of the steps outlined in this document serve as an extension of the [offsite payments extension tutorial](/docs/apps/build/payments/offsite/use-the-cli). To build a redeemables payments extension you will need to create a new app, with a redeemables payments extension and Checkout UI extension correctly applied. Create your new app using the [Shopify CLI](/docs/apps/build/scaffold-app). > Tip: > To create payment extensions via Shopify CLI you must be using `v3.60.0` or above. ## Step 2: Create a Checkout UI extension After creating your app, generate a Checkout UI extension and deploy your app to Shopify. This extension will be used to collect gift card details from buyers directly on checkout. 1. Use the Shopify CLI to [scaffold](/docs/api/checkout-ui-extensions#scaffolding-extension) a Checkout UI extension for your app. 2. Name your extension 3. Choose to work in TypeScript React. > Note: >A sample extension, written in Typescript React, is shown below in the Sample Checkout UI Extension section. 3. After you generate the extension, [deploy](/docs/api/shopify-cli/app/app-deploy) your app to [Shopify Partners](https://www.shopify.com/partners). This will allow you to link the Checkout UI extension with your payments app extension. 4. In Shopify Partners, click **Apps**. 5. Select your app. 6. Click **Extensions**. At this point, you should have the Checkout UI extension you created. 7. Click the name of your Checkout UI extension. 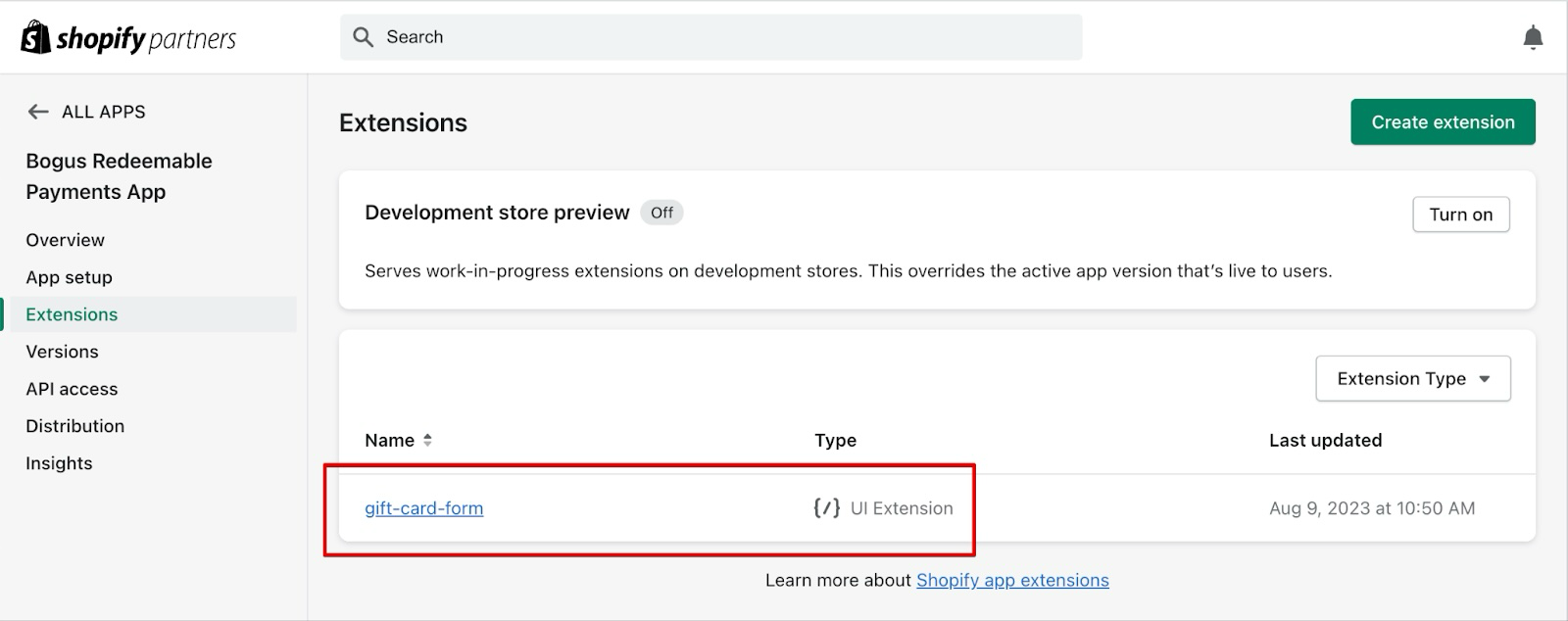 7. Follow this [guide](/docs/apps/launch/deployment/deploy-app-versions#create-and-release-an-app-version) to create and release a new version of your app. ## Step 3: Create a payments extension ### Generate the extension Your Shopify app becomes a payments app after you've created and configured your payments extension. **Redeemables** describes a payment method that can be redeemed. Examples are gift cards, store credit, etc. > Note: Shopify will need to enable the beta for your Shopify Partners account to see the redeemables payments extension. Shopify will also need to enable a beta for the shop you intend to test your payment method on. 1. Run the following command to start generating your payment extension: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script data-option="title" data-value="pnpm"></script> <script type="text/plain" data-language="bash"> RAW_MD_CONTENTpnpm shopify app generate extension END_RAW_MD_CONTENT</script> </div> </p> 1. When prompted, choose your organization & create this as a new app 1. When prompted for "Type of extension", select "Payments App Extension > Redeemable" and name your extension ### Set up your payments extension In this section, you will configure and deploy your application with the payments extension. 1. Disable embedding Shopify apps are embedded by default, but payments apps are an exception to this, because they don't need to render anything in Shopify admin. In `shopify.app.toml`, update the `embedded` and set it to false. 1. Configure basic app settings In `shopify.app.toml`, update the `name` and `client_id` to match the information about the app that you manually created. You can find the `client_id` in the **Client credentials** section of you app's overview page in the [Partner Dashboard](https://partners.shopify.com/apps/). 1. Push the configuration changes to your app and start your server In a terminal, run the following commands to push the configuration changes to your app: 1. Install the packages required to run the payments app: <p> <div class="react-code-block" data-preset="terminal"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar "></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="bash" data-title="npm"> RAW_MD_CONTENTnpm install END_RAW_MD_CONTENT</script> <script type="text/plain" data-language="bash" data-title="yarn"> RAW_MD_CONTENTyarn END_RAW_MD_CONTENT</script> <script type="text/plain" data-language="bash" data-title="pnpm"> RAW_MD_CONTENTpnpm install END_RAW_MD_CONTENT</script> </div> </p> 1. Deploy your app to update the config, which is defined in `shopify.app.toml`: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script data-option="title" data-value=""></script> <script type="text/plain" data-language="bash"> RAW_MD_CONTENTshopify app deploy END_RAW_MD_CONTENT</script> </div> </p> 1. Start your development server To run the app locally, start your development server: 1. <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script data-option="title" data-value=""></script> <script type="text/plain" data-language="bash"> RAW_MD_CONTENTshopify app dev END_RAW_MD_CONTENT</script> </div> </p> <Notice type="info"> You might be prompted to log in to your Partner account. </Notice> In your terminal, select your development store. You can use the generated URL to test your payments app by using it in your [payments app configuration](#step-4-configure-your-payments-extension). If you want a consistent tunnel URL, then you can use the `--tunnel-url` flag with your own tunnel when starting your server. 1. Press `p` to open the app in your browser. This brings you to your development store's admin, where you can install your payments app. ## Step 4: Configure your payments extension Configuration of a redeemables payments extension is similar to a [Offsite payments extension](/docs/apps/build/payments/offsite/use-the-cli). Your payments extension configures the following fields: | Property name | Description | | ------------- | ---------------------------------------------------------------------------------- | | `payment_session_url` <br /><span class="heading-flag">Required</span> | The URL that receives payment and order details from the checkout. | | `refund_session_url` <br /><span class="heading-flag">Required</span> | The URL that refund session requests are sent to. | | `capture_session_url` <br /><span class="heading-flag">Optional</span> | The URL that capture session requests are sent to. This is only used if your payments app supports merchant manual capture. | | `void_session_url` <br /><span class="heading-flag">Optional</span> | The URL that void session requests are sent to. This is only used if your payments app supports merchant manual capture or void payments. | | `supported_countries` <br /><span class="heading-flag">Required</span> | The countries where your payments app is available. Refer to the [list of ISO 3166 (alpha-2) country codes](https://www.iso.org/iso-3166-country-codes.html) where your app is available for installation by merchants. | | `supported_payment_methods` <br /><span class="heading-flag">Required</span> | The payment methods (for example, Visa) that are available with your payments app. [Learn more](https://github.com/activemerchant/payment_icons/blob/master/db/payment_icons.yml). | | `supports_installments` <br /><span class="heading-flag">Required</span> | Enables installments | | `supports_deferred_payments` <br /><span class="heading-flag">Required</span> | Enables deferred payments | | `merchant_label` <br /><span class="heading-flag">Optional</span> | The name for your payment provider extension. This name is displayed to merchants in the Shopify admin when they search for payment methods to add to their store. Limited to 50 characters. | | `buyer_label` <br /><span class="heading-flag">Optional</span> | The name of the method. Your checkout name can be the same as your merchant admin name or it can be customized for customers. This name is displayed with the payment methods that you support in the customer checkout. After a checkout name has been set, translations should be provided for localization. | | `test_mode_available` <br /><span class="heading-flag">Required</span> | Enables merchants using your payments app to test their setup by simulating transactions. To test your app on a development store, your payment provider in the Shopify admin must be set to test mode. | | `api_version` <br /><span class="heading-flag">Required</span> | The Payments Apps GraphQL API version used by the payment provider app to receive requests from Shopify. You must use the same API version for sending GraphQL requests. You can't use the unstable version of the API in production. API versions are updated in accordance with Shopify's general [API versioning timelines](/docs/api/usage/versioning). | | `multiple_capture` <br /><span class="heading-flag">Optional, Closed Beta</span> | Enables merchants using your payment provider app to partially capture an authorized payment multiple times up to the full authorization amount. This is used only if your payments app supports merchant manual capture. | | `balance_url` <br/><span class="heading-flag">Required</span> | The URL that balance requests are sent to. | | `ui_extension_handle` <br/><span class="heading-flag">Required</span> | The UI extension that will be used to render your payments app in checkout. This value can only be a UI extension linked to this specific payments app.| | `checkout_payment_method_fields` <br/><span class="heading-flag">Required</span> | The fields your payments app will accept from buyers in checkout (for example, installment details, payment plan). Each field is composed of a key name, as well as the data type, that restricts the input the buyer can provider. | > Note: > The `balance_url`, `ui_extension_handle`, and `checkout_payment_method_fields` are new app extension configurations, specifically for payments apps that process gift cards. You’ll want to select the UI extension you created in **Create a Checkout UI Extension** as the value for the `ui_extension_handle` field. The UI extension generated in [Create a Checkout UI extension](/docs/apps/build/payments/redeemables/build-a-redeemables-payment-extension#step-2-create-a-checkout-ui-extension) will determine what fields, validation, and form submission behavior is presented to buyers during checkout. ### UI Extension This is where you would identify the checkout extension you built prior to creating your payment extension to tie them into one another. | Property name | Description | | ------------- | ---------------------------------------------------------------------------------- | | `ui_extension_handle` <br/><span class="heading-flag">Required</span> | The UI extension that will be used to render your payments app in checkout. This value can only be a UI extension linked to this specific payments app.| ### UI Extension Field Definitions The fields you are looking to accept from your UI extensions so the payment method can validate the correct data is sent from the front end. | Property name | Description | | ------------- | ---------------------------------------------------------------------------------- | | `checkout_payment_method_fields` <br/><span class="heading-flag">Required</span> | The fields your payments app will accept from buyers in checkout (for example, installment details, payment plan). Each field is composed of a key name, as well as the data type, that restricts the input the buyer can provider. | ``` [[extensions.checkout_payment_method_fields]] key = "bank_name" type = "string" required = true [[extensions.checkout_payment_method_fields]] key = "account_number" type = "string" required = false ``` The above field definitions would be defined for something that looks like this: 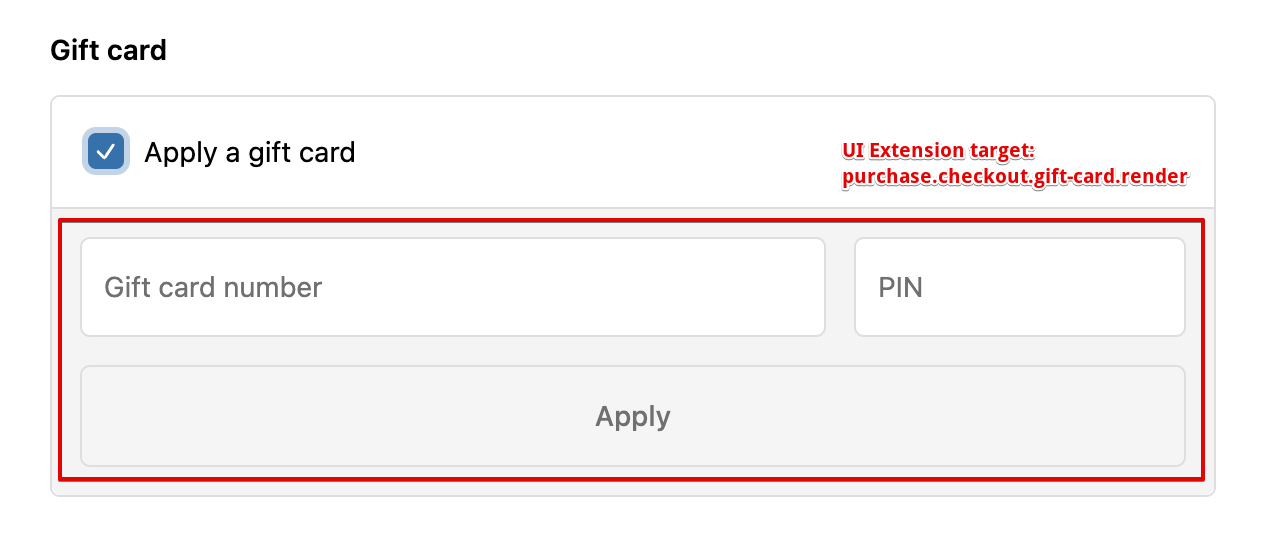 ## Step 5: Implement the Checkout UI extension Your UI extension must use the `purchase.checkout.gift-card.render` extension target. This ensures that your UI renders in the correct location for gift card payments and has access to the `applyRedeemableChange` API method to apply a gift card to a checkout. > Note: > The `purchase.checkout.gift-card.render` extension target is only available to beta Partners, and can only be used for payments apps that process gift cards. `applyRedeemableChange` is a new API method which can be pulled from the `useApplyRedeemableChange` hook on [@shopify/ui-extensions-react/checkout](https://www.npmjs.com/package/@shopify/ui-extensions-react). ### `applyRedeemableChange` This method applies a redeemable (i.e. gift card) to the checkout your extension is rendered on. This method triggers a *Fetch Balance* request explained later. The attributes provided to this method should mirror the definitions in the *UI Extension Field Definitions* configuration on your payments app extension. If the attributes provided don’t match your field definitions, the call will fail and an error will be returned. Example `applyRedeemableChange` payload: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="javascript"> RAW_MD_CONTENT{ type: 'redeemableAddChange' attributes: [ { 'key': 'card_number', 'value': '123456789' }, { 'key': 'pin', 'value': 1234 } ], identifier: '123456789' } END_RAW_MD_CONTENT</script> </div> </p> Example `applyRedeemableChange` usage: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="javascript"> RAW_MD_CONTENTimport { useApplyRedeemableChange, } from "@shopify/ui-extensions-react/checkout"; function Extension() { const applyRedeemableChange = useApplyRedeemableChange(); async function handleSubmit() { const change = { type: 'redeemableAddChange' attributes: [ { 'key': 'card_number', 'value': '123456789' }, { 'key': 'pin', 'value': 1234 } ], identifier: '123456789' } const result = await applyRedeemableChange(change); } // ... } END_RAW_MD_CONTENT</script> </div> </p> #### Success When applyRedeemableChange successfully completes, the following will be returned: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="javascript"> RAW_MD_CONTENT{ type: 'success' } END_RAW_MD_CONTENT</script> </div> </p> #### Error A number of errors may rise when `applyRedeemableChange` is run and fails. The output would look like: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="javascript"> RAW_MD_CONTENT{ type: 'error', message: 'Could not apply redeemable' } END_RAW_MD_CONTENT</script> </div> </p> `message` is human-readable, and can be any of: - Could not apply redeemable - Access denied: the extension does not have the required approval scopes - Invalid RedeemableChange type - Could not apply redeemable change: the buyer journey is completed These messages are for debugging and should not be displayed to the buyer. Shopify owns displaying errors to buyers within checkout. ### Example Refer to the [Sample Checkout UI extension](/docs/apps/build/payments/redeemables/build-a-redeemables-payment-extension#sample-checkout-ui-extension) for an example implementation of what your gift card form will look like. ### Things to consider While implementing the UI extension you may want to consider the following: - **Localization** - The labels and visible text displayed to the buyer should be localized. We provide the [useTranslate](/docs/apps/build/checkout/localized-checkout-ui-extensions/localize) hook through the StandardAPI for this purpose. - **Field validation** - Before making the `applyRedeemableChange` request, it is advisable to validate the gift card input fields. To present field validation messages, you can utilize the [error](/docs/api/checkout-ui-extensions/unstable/components/forms/textfield#textfieldprops-propertydetail-error) property provided by the TextField component. - **Loading, Error, and Success states** - When the buyer submits a gift card, your UI extension needs to handle loading, error, and success states. To accomplish this: - **Loading state**: Display a loading spinner in the “Apply” button while the request is pending. To achieve this, you can use the [Spinner](/docs/api/checkout-ui-extensions/unstable/components/feedback/spinner) component. - **Success state**: Reset the form fields to their initial state after successfully applying the gift card to the checkout. - **Error state**: Do not reset the form fields in case of an error. Instead, you may want to indicate which fields need to be corrected by utilizing field validation errors. - **Responsive UI** - Use [StyleHelper](/docs/api/checkout-ui-extensions/unstable/components/utilities/stylehelper) to make your UI extension responsive to different viewport sizes, focus states, and hover states. ## Implement the payments app Payments apps for gift cards are implemented very similarly to the existing [offsite payments apps](/docs/apps/build/payments/processing#offsite-payments). The key difference is that, for gift cards, payments apps have an operation that occurs prior to a checkout completing - fetching the balance of a gift card. ### Step 1: Fetch Balance The first step in a gift card payment is to retrieve the balance, so that it can be applied to a checkout. In order to do so, we require that you set up a **Balance URL** in the payments app extension. Shopify will make a request to that URL to retrieve the balance of a gift card during checkout. Example request payload: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="json"> RAW_MD_CONTENT{ "id": "uuid", "currency": "USD", "test": false, "merchant_locale": "en", "payment_method": { "type": "redeemable", "data": { "attributes": { "card_number": "123456789", "pin": 1234 } } } } END_RAW_MD_CONTENT</script> </div> </p> #### Request body <table id="request-attributes"> <tr> <th>Attribute</th> <th>Description</th> <th>Type</th> </tr> <tr> <td><code>id</code></td> <td>Globally unique identifier for the fetch balance attempt. Used as the <a href="/docs/apps/payments/implementation#idempotency">idempotency key</a>. Ensures that repeated requests with the same ID are treated the same, preventing duplicate fetch balance sessions.</td> <td><code>String</code></td> </tr> <tr> <td><code>currency</code></td> <td>Three-letter <a href="https://www.iso.org/iso-4217-currency-codes.html">ISO 4217 currency code</a>. For example <code>USD</code> or <code>CAD</code>.</td> <td><code>String</code></td> </tr> <tr> <td><code>test</code></td> <td>Indicates whether the payment is in <a href="/docs/apps/payments">test or live mode</a>.</td> <td><code>Boolean</code></td> </tr> <tr> <td><code>merchant_locale</code></td> <td> <a href="https://en.wikipedia.org/wiki/IETF_language_tag">IETF BCP 47 language tag</a> representing the language used by the merchant. For example, <code>en-US</code> or <code>fr-FR</code>. </td> <td><code>String</code></td> </tr> <tr> <td><code>payment_method</code></td> <td>Indicates the type and data relevant for the chosen payment method. Refer to <a href="#payment_method-hash">payment_method hash</a> for more information.</td> <td><code>Hash</code></td> </tr> </table> #### <code>payment_method</code> hash <table id="payment-method-hash"> <tr> <th>Attribute</th> <th>Description</th> <th>Type</th> </tr> <tr> <td><code>type</code></td> <td>Indicates the type of payment method. Currently only <code>"redeemable"</code> is supported.</td> <td><code>String</code></td> </tr> <tr> <td><code>data</code></td> <td>Contains data relevant for the chosen payment method. Refer to <a href="#payment_method-data-hash">payment_method.data hash</a> for more information.</td> <td><code>Hash</code></td> </tr> </table> #### <code>payment_method.data</code> hash <table id="payment-method-data-hash"> <tr> <th>Attribute</th> <th>Description</th> <th>Type</th> </tr> <tr> <td><code>attributes</code></td> <td>Contains the gift card details associated with the buyer. For example, <code>card_number</code> and <code>pin</code>. These fields are defined by the <a href="/docs/apps/build/payments/redeemables/build-a-redeemables-payment-extension#configure-your-payments-app-extension">UI Extension Field Definitions</a> configuration in your payments extension.</td> <td><code>String</code></td> </tr> </table> #### Success If the balance can be successfully fetched, send a HTTP 2xx response to the above request with a JSON body in the following format, including the balance: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="json"> RAW_MD_CONTENT{ "result": "success", "balance": { "value": "123.12", "currency": "CAD" } } END_RAW_MD_CONTENT</script> </div> </p> The provided value for `currency` can be any [ISO 4217](https://en.wikipedia.org/wiki/ISO_4217) currency code (i.e. USD, CAD). #### Failure In the event a balance fetch fails, there are a number of predefined codes you can respond with to surface an error to buyers. | Error Code | Description | | ------------- | ------------- | | REDEEMABLE_INVALID | The redeemable’s parameters are invalid. This is a generic error response.| | REDEEMABLE_INSUFFICIENT_BALANCE | The redeemable has insufficient balance.| | REDEEMABLE_EXPIRED | The redeemable has expired.| | REDEEMABLE_DISABLED | The redeemable was disabled.| Example failure response (HTTP 2xx): <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="javascript"> RAW_MD_CONTENT{ 'result': 'failure', 'reason': 'REDEEMABLE_INSUFFICIENT_BALANCE', 'message': 'The redeemable has insufficient funds.', } END_RAW_MD_CONTENT</script> </div> </p> #### Fetch balance sequence diagram 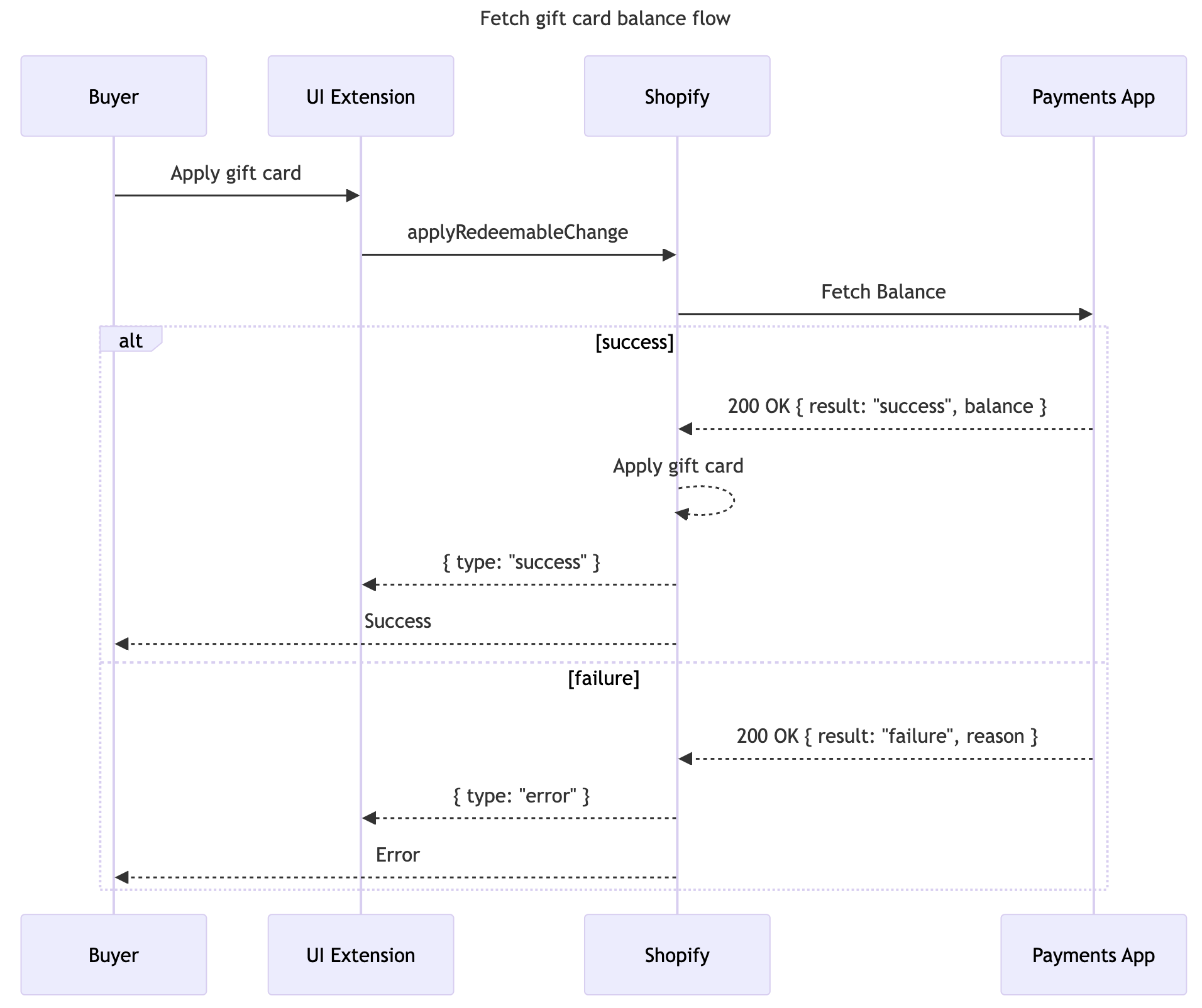 1. The buyer enters their gift card details into the UI Extension. 2. The UI extension, with the gift card details, calls `applyRedeemableChange`, available through [@shopify/ui-extensions-react/checkout](https://www.npmjs.com/package/@shopify/ui-extensions-react). 3. Shopify will call the payments app’s endpoint located at their configured **Balance URL**, with a request to fetch the balance of the provided gift card. 4. The payments app returns the balance for the gift card. 5. Shopify applies the gift card to the buyer’s checkout. 6. The buyer is shown that the gift card has been applied to their checkout. ### Step 2: Process Payment 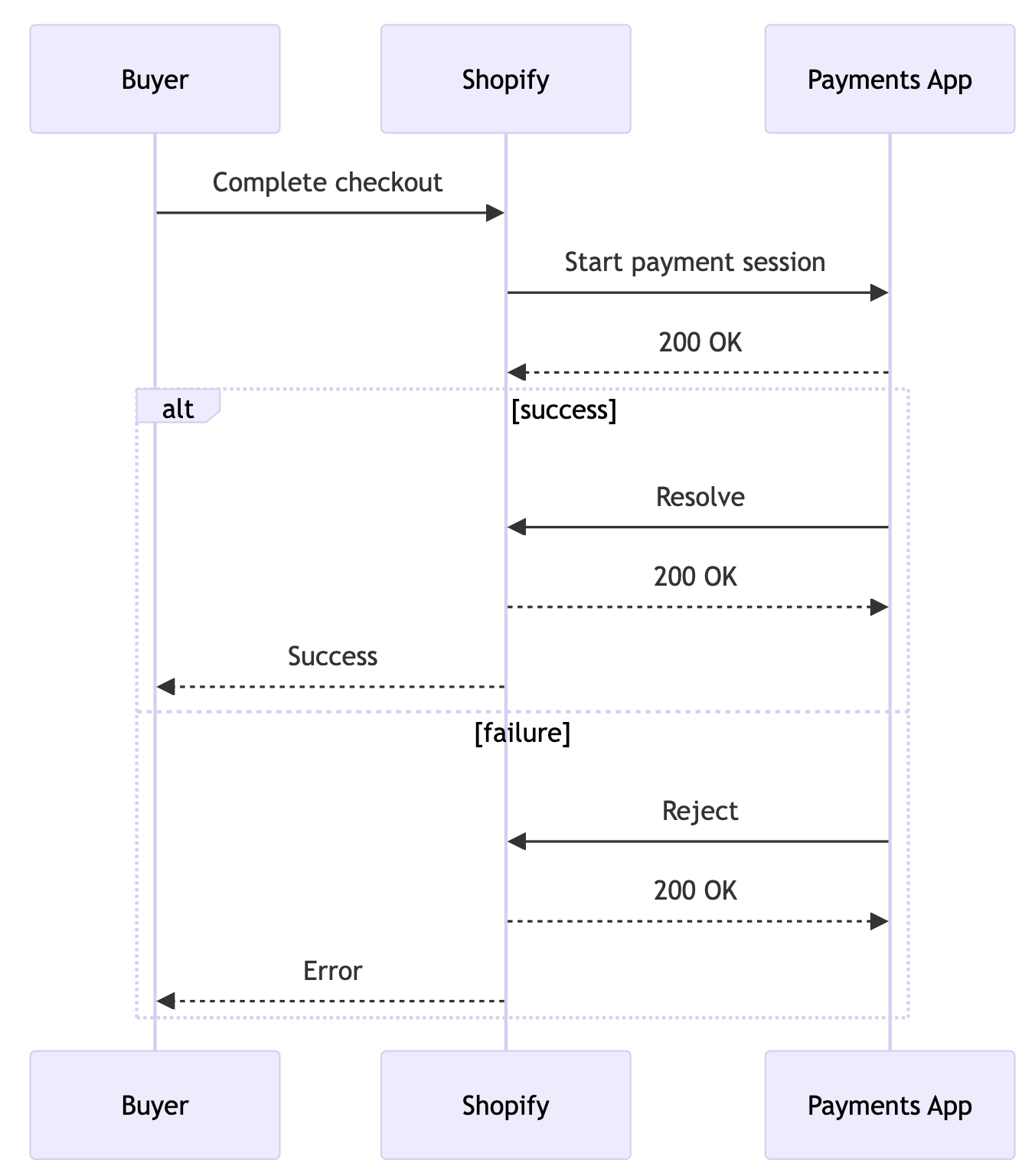 Payments with payments apps are processed asynchronously. When the buyer completes their checkout, a request will be sent from Shopify to the *Payment session URL* defined in [Configure your payments app extension](/docs/apps/build/payments/redeemables/build-a-redeemables-payment-extension#step-4-configure-your-payments-extension), with the checkout and payment details. The Payments app should respond with HTTP 2xx to indicate that the payment session was started, and should begin processing the gift card payment at this point. Example request body: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="json"> RAW_MD_CONTENT{ "id": "u0nwmSrNntjIWozmNslK5Tlq", "gid": "gid://shopify/PaymentSession/u0nwmSrNntjIWozmNslK5Tlq", "group": "rZNvy+1jH6Z+BcPqA5U5BSIcnUavBha3C63xBalm+xE=", "session_id": "4B2dxmle3vGgimS4deUX3+2PgLF2+/0ZWnNsNSZcgdU=", "amount": "123.00", "currency": "CAD", "test": false, "merchant_locale": "en", "payment_method": { "type": "redeemable", "data": { "attributes" : { "card_number": "123456789", "pin": 1234 }, }, }, "proposed_at": "2020-07-13T00:00:00Z", "customer": { "shipping_address": { "given_name": "Alice", "family_name": "Smith", "line1": "123 Street", "line2": "Suite B", "city": "Montreal", "postal_code": "H2Z 0B3", "province": "Quebec", "country_code": "CA", "phone_number": "5555555555" }, "email": "buyer@example.com", "phone_number": "5555555555", "locale": "fr" }, "kind": "sale" } END_RAW_MD_CONTENT</script> </div> </p> Details on each attribute can be found [here](/docs/apps/build/payments/request-reference#request-body). The payment method data object in the payment session request body is defined by the aforementioned *UI Extension Field Definitions* configuration in your app extension, just like for Fetch Balance. If the request fails, then it's retried several times. If the request still fails, then the customer needs to retry their payment through Shopify checkout. If there's an error on the payments app's side, then don't respond with an HTTP 2xx. Use an appropriate error status code instead. #### Resolve Once the payments app has responded to the initial start payment session request, it should begin processing the payment. Since this is an asynchronous process, the payments app will be performing the next step independently, through the [paymentSessionResolve](/docs/api/payments-apps/2023-07/mutations/paymentSessionResolve) mutation on the [Payments Apps GraphQL API](/docs/api/payments-apps). This mutation will resolve the payment session, indicating that the payment was successful. Example GraphQL mutation: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="graphql"> RAW_MD_CONTENTmutation PaymentSessionResolve($id: ID!) { paymentSessionResolve(id: $id) { paymentSession { id state { ... on PaymentSessionStateResolved { code } } } userErrors { field message } } } END_RAW_MD_CONTENT</script> </div> </p> With this input: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="json"> RAW_MD_CONTENT{ "id": "gid://shopify/PaymentSession/u0nwmSrNntjIWozmNslK5Tlq" } END_RAW_MD_CONTENT</script> </div> </p> Example JSON response: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="json"> RAW_MD_CONTENT{ "data": { "paymentSessionResolve": { "paymentSession": { "id": "gid://shopify/PaymentSession/u0nwmSrNntjIWozmNslK5Tlq", "state": { "code": "RESOLVED" } }, "userErrors": [] } } } END_RAW_MD_CONTENT</script> </div> </p> After this, the payment will be marked as resolved in Shopify. #### Reject If a payment was unsuccessful for any reason, the payments app must use the [paymentSessionReject](/docs/api/payments-apps/2023-07/mutations/paymentSessionReject) mutation. Example GraphQL mutation: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="graphql"> RAW_MD_CONTENTmutation PaymentSessionReject( $id: ID!, $reason: PaymentSessionRejectionReasonInput! ) { paymentSessionReject( id: $id, reason: $reason ) { paymentSession { id state { ... on PaymentSessionStateRejected { code reason merchantMessage } } } userErrors { field message } } } END_RAW_MD_CONTENT</script> </div> </p> With this input: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="json"> RAW_MD_CONTENT{ "id": "gid://shopify/PaymentSession/u0nwmSrNntjIWozmNslK5Tlq", "reason": { "code": "PROCESSING_ERROR", "merchantMessage": "the payment didn't work" } } END_RAW_MD_CONTENT</script> </div> </p> Example JSON response: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="json"> RAW_MD_CONTENT{ "data": { "paymentSessionReject": { "paymentSession": { "id": "gid://shopify/PaymentSession/u0nwmSrNntjIWozmNslK5Tlq", "state": { "code": "REJECTED", "reason": "PROCESSING_ERROR", "merchantMessage": "the payment didn't work" }, }, "userErrors": [] } } } END_RAW_MD_CONTENT</script> </div> </p> #### Error There are some defined errors that you can reject a paymentSession with. | Error Code | Description | | ------------- | ------------- | | PROCESSING_ERROR | There was an error processing the payment. | ### Step 3: Refunds Refunds operate the same as they do with regular payments. Documentation can be found [here](/docs/apps/build/payments/processing#refunds). ### Optional: Authorizations, Captures, and Voids Authorizations, captures, and voids allow merchants to use your payment app to initiate separate transactions to authorization and capture payments. These will operate the same as they do with regular payment apps. More context about authorizations can be found [here](https://help.shopify.com/en/manual/payments/payment-authorization#set-up-manual-capture-of-credit-card-payments). Merchants control whether or not they use "sale or "authorization" payment transactions by changing their *Payment capture method* payment setting to "Manual" or "Automatically when order is fulfilled". For details on how to implement and respond to capture and void session requests see: | | Diagram | Documentation | | ------------- | ------------- | ----------- | | **Captures** | [Capture overview](/docs/apps/build/payments/processing#captures) | [Explore capture sessions](/docs/apps/build/payments/offsite/use-the-cli#explore-capture-sessions-(optional)) | | **Voids** | [Void overview](/docs/apps/build/payments/processing#voids) | [Explore void sessions](/docs/apps/build/payments/offsite/use-the-cli#explore-void-sessions-(optional)) | ## Sample Checkout UI Extension Use the following example for implementing your gift card form: <p> <div class="react-code-block" data-preset="basic"> <div class="react-code-block-preload ThemeMode-dim"> <div class="react-code-block-preload-bar basic-codeblock"></div> <div class="react-code-block-preload-placeholder-container"> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> <div class="react-code-block-preload-code-container"> <div class="react-code-block-preload-codeline-number"></div> <div class="react-code-block-preload-codeline"></div> </div> </div> </div> <script type="text/plain" data-language="tsx"> RAW_MD_CONTENTimport { BlockStack, Button, Form, Grid, reactExtension, Style, TextField, useApplyRedeemableChange, } from "@shopify/ui-extensions-react/checkout"; import { RedeemableChange } from "@shopify/ui-extensions/checkout"; import { useState, useCallback } from "react"; export default reactExtension("purchase.checkout.gift-card.render", () => ( <Extension /> )); function Extension() { const [cardNumber, setCardNumber] = useState(""); const [pin, setPin] = useState(""); const [loading, setLoading] = useState(false); const applyRedeemableChange = useApplyRedeemableChange(); async function handleSubmit() { setLoading(true); const change = { type: "redeemableAddChange", attributes: [ { key: "card_number", value: cardNumber }, { key: "pin", value: pin }, ], identifier: cardNumber, } as RedeemableChange; try { const result = await applyRedeemableChange(change); if (result.type === "success") { setCardNumber(""); setPin(""); } else { console.log(result.message); } } finally { setLoading(false); } } const isFormValid = cardNumber.length > 0 && pin.length > 0; return ( <Form onSubmit={handleSubmit}> <BlockStack> <Grid columns={Style.default("fill").when( { viewportInlineSize: { min: "small" } }, ["70%", "30%"] )} spacing="base" > <TextField label="Gift card number" value={cardNumber} onInput={useCallback( (newValue: string) => setCardNumber(newValue), [] )} /> <TextField label="PIN" value={pin} onInput={useCallback((newValue: string) => setPin(newValue), [])} /> </Grid> <Button accessibilityRole="submit" disabled={!isFormValid} loading={loading} > Apply </Button> </BlockStack> </Form> ); } END_RAW_MD_CONTENT</script> </div> </p> ## Tutorial Complete Congratulations! You set up a redeemable payments extension.